This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
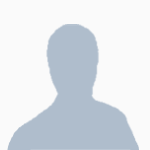
Need help with blackjack coding
Started by Zombie_brine, 17 September 2012 - 07:36 PMPosted 17 September 2012 - 09:36 PM
I need some code that would output a random number of redstone pulses between 1-13. Ill credit you in the video i will eventually make.
Posted 17 September 2012 - 10:00 PM
You can use math.random(1,13) –1 being the start point, 13 being the end point.
Implemented like this:
Implemented like this:
local pulse = math.random(1,13)
for i = 1,#pulse do
rs.setOutput("side",true)
sleep(.5)
rs.setOutput("side",false)
end
Posted 17 September 2012 - 11:26 PM
Don't forget to add a sleep after setting the output to false, or it won't work. Also, pulses is a number, so you can't use the lenght operator on it.You can use math.random(1,13) –1 being the start point, 13 being the end point.
Implemented like this:local pulse = math.random(1,13) for i = 1,#pulse do rs.setOutput("side",true) sleep(.5) rs.setOutput("side",false) end
local pulses = math.random(1, 13)
for i = 1, pulses do
rs.setOutput("side", true)
sleep(0.2)
rs.setOutput("side", false)
sleep(0.2)
end
Posted 17 September 2012 - 11:35 PM
Ah yes, you are right. I am so used to working with tables on the for loops, I didn't even think about that. Also, you wouldn't need to add the sleep after false, because once it finishes, it will restart the loop. So unless he is wanting something to stay off for a long period of time, he can leave the second sleep() out of there. It's an either/or situation.
Posted 17 September 2012 - 11:38 PM
Actually, if you don't put the second sleep it won't make pulses, because it will turn it on, wait some time, turn it off and immediately turn it on, so the redstone is not updated and there's no pulse, just a continuous redstone signal.
Posted 17 September 2012 - 11:41 PM
Stop making arguments with LOGIC!!!! You're right, I'm wrong. Haha, I am not on a roll today. :)/>/>
Posted 18 September 2012 - 12:01 AM
whenever i try this i get
"deal:2: attempt to get length of number"
as an error
"deal:2: attempt to get length of number"
as an error
Posted 18 September 2012 - 12:13 AM
Read MysticT's post. He is correct on this one. I was going from a slow work day, not thinking. Ha…
Posted 18 September 2012 - 12:43 AM
coppied code from mystic verbatum -
"deal:3: bad argument: String expected, got nil"
Ideas..
"deal:3: bad argument: String expected, got nil"
Ideas..
Posted 18 September 2012 - 01:30 AM
Are you sure it was verbatim? Sounds like you did:
…instead of :
Or whatever the side was.
rs.setOutput(back, true)
…instead of :
rs.setOutput("back", true)
Or whatever the side was.
Posted 18 September 2012 - 02:54 AM
I had to rethink the system.. how would i make it 1-10 but have 10 in the pool 4 times (10,j,q,k)
Posted 18 September 2012 - 03:08 AM
"I had to rethink the system.. how would i make it 1-10 but have 10 in the pool 4 times (10,j,q,k)"
Not sure if this would work but…
local pulse = math.random(1,10,10,10,10)
Would this have the desired effect?
Not sure if this would work but…
local pulse = math.random(1,10,10,10,10)
Would this have the desired effect?
Posted 18 September 2012 - 05:02 AM
nope, but this would
local numPulses = math.min(math.random(1,13),10)
min() returns the smallest of it's params. 1-10 are unchanged, 11-13 are changed to 10.
local numPulses = math.min(math.random(1,13),10)
min() returns the smallest of it's params. 1-10 are unchanged, 11-13 are changed to 10.
Posted 18 September 2012 - 06:52 AM
Erm….
local pulses = math.random(1, 13)
Get rid of the space.
local pulses = math.random(1,13)
I think?Posted 18 September 2012 - 02:39 PM
Erm….Get rid of the space.local pulses = math.random(1, 13)
I think?local pulses = math.random(1,13)
The space is fine. Spacing arguments out can help improve readability.
Posted 19 September 2012 - 03:00 AM
replaced the line, "deal :2: "for" limit must be a number"nope, but this would
local numPulses = math.min(math.random(1,13),10)
min() returns the smallest of it's params. 1-10 are unchanged, 11-13 are changed to 10.
Worked before i changed just that line…
Posted 19 September 2012 - 03:47 PM
That new line has numPulses as the name of the variable, the loops mentioned earlier use pulses as the name. Ensure that they match and that error should go away.