local function uninstall(directory)
for i,v in ipairs(fs.list(directory)) do
fs.delete(v)
end
end
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
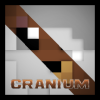
[Help] Uninstall function
Started by Cranium, 25 September 2012 - 12:33 AMPosted 25 September 2012 - 02:33 AM
I am trying to write an uninstall function into one of my systems, so instead of telling it to delete all of the files individually, I want it to delete them all in one pass. I know I can use a for loop to accomplish this, but it is not working. Can anyone tell me why? I do use ".MCS" as my directory name, if that means anything.
Posted 25 September 2012 - 04:23 AM
It should work.. Just make sure the directory name is in "quotes"
Posted 25 September 2012 - 01:56 PM
To delete an entire directory with files and other directories inside:
If there are files in lets say the root folder of the computer or disk (Not in a folder/directory) then you might be able to make a table containing the file's names and stuff. Otherwise I think you will have to add something like this:
If this doesn't work for you then sorry! But it has worked for me so I would expect it to work for you.
ALSO: If you were referring to deleting all the files inside the folder without deleting the folder, I don't know how to do that without deleting it, BUT you COULD do this:
fs.delete("[directory]")
OR to delete a directory inside a directory:
fs.delete("[directory/directory]")
If there are files in lets say the root folder of the computer or disk (Not in a folder/directory) then you might be able to make a table containing the file's names and stuff. Otherwise I think you will have to add something like this:
fs.delete("[file1]")
fs.delete("[file2]")
If this doesn't work for you then sorry! But it has worked for me so I would expect it to work for you.
ALSO: If you were referring to deleting all the files inside the folder without deleting the folder, I don't know how to do that without deleting it, BUT you COULD do this:
fs.delete("[directory]")
fs.makeDir("[directory]")
Which should delete all the files inside and recreate the directory.Posted 25 September 2012 - 05:44 PM
I tried that, I just made the function call as uninstall(".MCS"), and nothing happened. The files were still there.It should work.. Just make sure the directory name is in "quotes"
And deleting the directory did not work, the directory was still there, as well as the files. The only reason I want to make an iteration like this, would be to install a program pack, then at request, be able to uninstall it all at once, but without specifying each and every file. That way, I can update the program pack as neccessary(probably through the HTTP API), and just have the uninstall be general so it can uninstall everytthing in the root directory of the program pack.ALSO: If you were referring to deleting all the files inside the folder without deleting the folder, I don't know how to do that without deleting it, BUT you COULD do this:Which should delete all the files inside and recreate the directory.fs.delete("[directory]") fs.makeDir("[directory]")
Posted 25 September 2012 - 10:47 PM
I tried that, I just made the function call as uninstall(".MCS"), and nothing happened. The files were still there.It should work.. Just make sure the directory name is in "quotes"And deleting the directory did not work, the directory was still there, as well as the files. The only reason I want to make an iteration like this, would be to install a program pack, then at request, be able to uninstall it all at once, but without specifying each and every file. That way, I can update the program pack as neccessary(probably through the HTTP API), and just have the uninstall be general so it can uninstall everytthing in the root directory of the program pack.ALSO: If you were referring to deleting all the files inside the folder without deleting the folder, I don't know how to do that without deleting it, BUT you COULD do this:Which should delete all the files inside and recreate the directory.fs.delete("[directory]") fs.makeDir("[directory]")
That's weird that it does not work for you, as it works for me! :/
Posted 25 September 2012 - 10:53 PM
I'll try again when I get home after work. If it's supposed to work, then there may be something wrong with the CCemulator I use. I'll try it in CCemu and in minecraft to see if that makes a change.
Posted 25 September 2012 - 11:00 PM
Are you getting the / in front of the dir? This is the syntax that I have been using and it removes the dir and all the files.
fs.delete("/DirName")
Posted 25 September 2012 - 11:07 PM
No, I'm not. Maybe that is what's wrong. But if I use the fs.list(".MCS"), it would return all files within that directory. It doesn't change if I use the "/" or not. I did test a few things.
for i,v in ipairs(fs.list(".MCS")) do --tried ipairs
fs.delete(v)
end
for i,v in pairs(fs.list(".MCS")) do --tried pairs
fs.delete(v)
end
for i,v in pairs(fs.list(".MCS")) do
print(v) --it printed all the files listed in /.MCS
end
I tried all of those loops, but it never did delete the files, but it would print the filenames. I wonder why that is?Posted 25 September 2012 - 11:52 PM
Ok, the fs.delete("directory") should work. But if you still want to delete the files without removing the folder, then you can use the loop with fs.list, but fs.list returns the names of the files/directories inside the specified path, not the whole path, and fs.delete needs the whole path. So, you need to combine the given path with the file name:
local function uninstall(path)
for _,file in ipairs(fs.list(path)) do
fs.delete(fs.combine(path, file))
end
end
Posted 26 September 2012 - 12:03 AM
local function uninstall(path)
for i,v in ipairs(fs.list(path)) do
fs.delete(fs.combine(path, v))
end
end
uninstall(".MCS")
So that would work?^Posted 26 September 2012 - 12:08 AM
Yes, that should work.
Posted 26 September 2012 - 03:47 AM
Weird thing to ask here, but. What does "For i, v, in ipairs" do?Ok, the fs.delete("directory") should work. But if you still want to delete the files without removing the folder, then you can use the loop with fs.list, but fs.list returns the names of the files/directories inside the specified path, not the whole path, and fs.delete needs the whole path. So, you need to combine the given path with the file name:local function uninstall(path) for _,file in ipairs(fs.list(path)) do fs.delete(fs.combine(path, file)) end end
Posted 26 September 2012 - 06:41 AM
http://lua-users.org/wiki/ForTutorial
For is a loop. You will find info about your question in this link
For is a loop. You will find info about your question in this link