This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
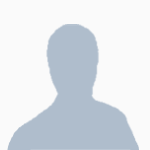
Precise Turtle Bomber help
Started by Jellydonut78, 08 October 2012 - 10:59 PMPosted 09 October 2012 - 12:59 AM
I am on a multiplayer tekkit server so i cant access the files to copy and paste in, so dont just give me a link. I made a program on a turtle to strafe run and drop tnt for 16 blocks and return. I really want to be able to just say bomb (x) (y) (z) and have it go drop a bomb and return. My current program has a password lock using local answers and all that good stuff, so im not that new to lua. I also dont want to have 4 computers with gps set up every hundred or so blocks so a memory file would be needed. Please help me do this so i can stick it to those griefing players. (:
Posted 09 October 2012 - 01:25 AM
My moveTo function often works like this:
Change the value of x,y and z at the top of the code (by reading it from the user with read() for example). Then call moveTo(1,2,3) to move your turtle to 1,2,3 : ) Code is untested due to not having CC here >.<.
local x,y,z = 0,0,0
local orientation = 0
local function round(n)
return math.floor( n + 0.5 )
end
local function forward(n)
for i=1,n do
while not turtle.forward() do
turtle.dig()
end
x = x + round(math.cos(orientation*(math.pi/2)))
z = z + round(math.sin(orientation*(math.pi/2)))
end
end
local function turnRight(n)
n = n or 1
for i=1,n do
orientation = (orientation - 1)%4
return turtle.turnRight()
end
end
local function turnLeft(n)
n = n or 1
for i=1,n do
orientation = (orientation + 1)%4
return turtle.turnLeft()
end
end
local function turnTo(o)
while orientation ~= o do
turnRight()
end
end
local function vertical(y_i)
if (y_i < y) then
for i=1,(y-y_i) do
while not turtle.down() do turtle.digDown() end
end
elseif (y_i > y) then
for i=1,(y_i-y) do
while not turtle.up() do turtle.digUp() end
end
end
end
local function moveTo(xi,yi,zi)
if (x_i > x) then
turnTo(0)
forward(x_i-x)
elseif (x_i < x) then
turnTo(2)
forward(x_i-x)
end
if (z_i > z) then
turnTo(1)
forward(z_i-z)
elseif (z_i < z) then
turnTo(2)
forward(z_i-z)
end
vertical(yi)
end
Change the value of x,y and z at the top of the code (by reading it from the user with read() for example). Then call moveTo(1,2,3) to move your turtle to 1,2,3 : ) Code is untested due to not having CC here >.<.
Posted 09 October 2012 - 01:32 AM
Damn, Orkwell beat me.
Posting my variation anyways :D/>/>
Posting my variation anyways :D/>/>
local args = {
...
}
if not #args == 4 then
print('Usage: bomb <x> <y> <z>')
end
local x, y, z = args[1], args[2], args[3]
for i = 1, x do
turtle.forward()
end
for i = 1, y do
turtle.up()
end
if z < 0 then
turtle.turnLeft()
else
turtle.turnRight()
end
for i = 1, z do
turtle.forward()
end
rs.setOutput('bottom', true)
turtle.select(1)
turtle.placeDown()
turtle.turnLeft()
turtle.turnLeft()
for i = 1, z do
turtle.forward()
end
if z < 0 then
turtle.turnRight()
else
turtle.turnLeft()
end
for i = 1, y do
turtle.down()
end
for i = 1, x do
turtle.forward()
end
Posted 09 October 2012 - 01:43 AM
Kingdaro, Your code looks promising, but i am ona server using computercraft 1.3 i think. So there is no fuel to check. If you could update the code i would try it. Your code is by far the easiest one out ther at this point in time.
Posted 09 October 2012 - 01:43 AM
Fuel… I forgot about fuel. : ) And due to the short life time of the turtle, my whole calculations for orientation are redundant, so you might wanna use Kingdaro's version. ; )
Posted 09 October 2012 - 01:49 AM
Edited my original post without fuel checks.
Posted 09 October 2012 - 01:55 AM
kingdaro, could you also tell me how to use the program too. like do i change some values inside it or somthing? Im not good at looking at code and figuring that out.
Posted 09 October 2012 - 02:07 AM
…That's sort of a predicament, actually. I don't think the pastebin API exists in tekkit, so I think the only way would be to type it in word for word.
After you've done that, though, you can just type in the command prompt "bomb <x> <y> <z>", with your TNT in the first slot.
Also, not that my code is basically completely untested, so keep that in mind. :D/>/>
After you've done that, though, you can just type in the command prompt "bomb <x> <y> <z>", with your TNT in the first slot.
Also, not that my code is basically completely untested, so keep that in mind. :D/>/>
Posted 09 October 2012 - 02:15 AM
Ok, but currently as is i think it has to be launched fro coords 0,0. I think it needs to be programmed with its current coordinates
Posted 09 October 2012 - 02:36 AM
i am getting an error for the for i = 1 telling me it must be a number.
could you test this program and see if you can get it to work, i am having trouble with it
could you test this program and see if you can get it to work, i am having trouble with it
Posted 09 October 2012 - 02:57 AM
Alright, I debugged it. Here's the revised code.
The X value is the amount of times forward, the Y value is the amount of times up, and the Z value is right if positive, and left if negative.
You could make it go backwards if the X value was negative, and down if the Y value was negative, but I don't think it's worth the effort, personally.
It, by default, starts at 0,0,0.Ok, but currently as is i think it has to be launched fro coords 0,0. I think it needs to be programmed with its current coordinates
The X value is the amount of times forward, the Y value is the amount of times up, and the Z value is right if positive, and left if negative.
You could make it go backwards if the X value was negative, and down if the Y value was negative, but I don't think it's worth the effort, personally.
Posted 09 October 2012 - 01:03 PM
could i change numbers to make it the current game coords and then give other coordinates and have it go?
Posted 09 October 2012 - 01:09 PM
I am going to make some functions inside of this program that will give it its current x y z coords when you manually input them. Then make more functions that auto update those coordinates. Thanks everyone you were ahuge help. (:
Jellydonut78
Jellydonut78
Posted 09 October 2012 - 10:16 PM
I am getting no errors now but the turtle goes up like 30 blocks and then stops. nothing else happens.