I'd like a program I'm currently writing to run a different program of mine (Such as a program created entirely for changing global variables using user input) if a certain "if" criteria is met. I've looked around, but can't seem to find any way to do this besides copy-pasting the entire program into the original script. Any help?
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
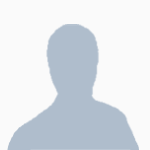
Run a program from inside another program (Like Inception, but with less DiCaprio)
Started by Datashocker, 07 March 2012 - 05:29 PMPosted 07 March 2012 - 06:29 PM
Hey there, ComputerCraft pros. As always, I apologize if this is painstakingly obvious. I'm new to coding.
I'd like a program I'm currently writing to run a different program of mine (Such as a program created entirely for changing global variables using user input) if a certain "if" criteria is met. I've looked around, but can't seem to find any way to do this besides copy-pasting the entire program into the original script. Any help?
I'd like a program I'm currently writing to run a different program of mine (Such as a program created entirely for changing global variables using user input) if a certain "if" criteria is met. I've looked around, but can't seem to find any way to do this besides copy-pasting the entire program into the original script. Any help?
Posted 07 March 2012 - 06:58 PM
that's pretty simple, actually, just:
shell.run(programpath)
shell.run(programpath)
Posted 07 March 2012 - 07:05 PM
One thing I know how to do is you can use
os.loadAPI("filegoeshere")
and from there you can call functions inside of that, with:
filegoeshere.myfunction()
So if the part you want to run is a function, that should work. (you can make the whole thing a function, and after you have the function part, make it run the function so that it acts the same)
There are probably other ways using the shell.run command, but I'm not sure how.
EDIT:
ninja'd
os.loadAPI("filegoeshere")
and from there you can call functions inside of that, with:
filegoeshere.myfunction()
So if the part you want to run is a function, that should work. (you can make the whole thing a function, and after you have the function part, make it run the function so that it acts the same)
There are probably other ways using the shell.run command, but I'm not sure how.
EDIT:
ninja'd
Posted 07 March 2012 - 07:17 PM
2 things:
- You can use:
- If you want to save variables and get accsess on them you could create a .txt file
that gets written with your variables!
I used this on my 'Mine-menu' program to save settings and get accsess on them from other
programs!
Use those functions to save:
And those to load:
Hope i helped!
-Zitrone77
- You can use:
shell.run("/YOUR-PROGRAM-PATH")
Whis will start 'YOUR-PROGRAM' and then continue when 'YOUR-PROGRAM' ends.- If you want to save variables and get accsess on them you could create a .txt file
that gets written with your variables!
I used this on my 'Mine-menu' program to save settings and get accsess on them from other
programs!
Use those functions to save:
file = io.open("/YOUR-SETTIGS-FILE.txt-PATH") -- opens 'YOUR-SETTINGS-FILE.txt'
file:write(YOUR-VARIABLE.."n") -- writes 'YOUR-VARIABLE' in the first line of 'YOUR-SETTINGS-FILE.txt'
file:wirte(YOUR-VARIABLE2.."n") -- writes 'YOUR-VARIABLE2' in the second line of 'YOUR-SETTINGS-FILE.txt'
file:close()And those to load:
file = io.open("/YOUR-SETTIGS-FILE.txt-PATH") -- opens 'YOUR-SETTINGS-FILE.txt'
YOUR-VARIABLE-OUT = file:read()
YOUR-VARIABLE2-OUT = file:read()
file:close
Hope i helped!
-Zitrone77
Posted 10 March 2012 - 07:53 PM
Thanks for the help, everyone. I've got my program working now.
Posted 31 May 2012 - 09:39 AM
so, for examplem, i can create a file caled "fil" with function "func" and then within another program get results of this function with fil.func(a,:)/>/> and those a,b it takes from current program?
Posted 31 May 2012 - 10:24 AM
so, for examplem, i can create a file caled "fil" with function "func" and then within another program get results of this function with fil.func(a, :)/>/> and those a,b it takes from current program?
You could use the os.loadAPI() function, but personally I prefer global tables, it's simple and flexible
add = {}
function add.one( num )
return num+1
end
function add.two( num )
return num+2
end
Edit: Don't use local on those function declarations, it'll be local if the table is
Posted 31 May 2012 - 06:19 PM
I don't like using global variables, and I don't think it's the best for that.so, for examplem, i can create a file caled "fil" with function "func" and then within another program get results of this function with fil.func(a, :)/>/> and those a,b it takes from current program?
You could use the os.loadAPI() function, but personally I prefer global tables, it's simple and flexibleadd = {} function add.one( num ) return num+1 end function add.two( num ) return num+2 end
Edit: Don't use local on those function declarations, it'll be local if the table is
If you have more than one function, you can make it an api an load it with os.pullEvent and the use it like any other api.
If you just need to use one function, just make the whole program the function, and give the parameters through shell.run().
Example:
File sum:
local tArgs = { ... } -- get the given arguments
local n = 0
for i = 1, #tArgs do
n = n + tonumber(tArgs[i])
end
return n
Your program:
local ok, n = shell.run("sum", "10", "15") -- run the sum program
if ok then -- check if the program had any errors
print(n) -- print the returned value
else
print("Error: ", n) -- there was an error in the program
end
Posted 31 May 2012 - 09:11 PM
I don't like using global variables, and I don't think it's the best for that.
API:
table.function() –Global
Global table:
table.function() –Global
There's no significant difference, it's a matter of preference :)/>/>
Just like the difference between
function Name() end
and
Name = function() end
Besides, the implementation of Lua I'm more familiar with doesn't have APIs at all, so it's certainly not detrimental to have global tables with functions
It's certainly better than having global functions on their own :)/>/>
Posted 31 May 2012 - 09:24 PM
Yes, I know it's the same, but what I mean is that you shouldn't rely on a global variable on another program, since it might not be what you expect it to be. I wouldn't either use shell.run if I can just place the functions inside the program.
The problem with global variables is that they can mess up other programs, since they all run in the same environment, so when you define a global variable in your program, other programs can access that (intentionally or not). I've seen programs that stop working just because of this.
The problem with global variables is that they can mess up other programs, since they all run in the same environment, so when you define a global variable in your program, other programs can access that (intentionally or not). I've seen programs that stop working just because of this.
Posted 31 May 2012 - 10:00 PM
Yes, I know it's the same, but what I mean is that you shouldn't rely on a global variable on another program, since it might not be what you expect it to be. I wouldn't either use shell.run if I can just place the functions inside the program.
The problem with global variables is that they can mess up other programs, since they all run in the same environment, so when you define a global variable in your program, other programs can access that (intentionally or not). I've seen programs that stop working just because of this.
If you follow the best practices, that really won't be an issue
Use local when possible, unique and descriptive variables, and validate everything before it's used :P
Global variables and variables from APIs are modified in the same way, so there is really no difference
If someone was making a global called "help",
help = "Help me!"
and suddenly your help API is goneHow is that superior, then? It's the same thing :P
The only protection APIs offer is that their metatables are protected against edits, which means nothing since trying to edit a nil would error anyway, every working script will have a declaration beforehand :P
As for not relying on another program… That is, again, the exact same as an API :p
Posted 31 May 2012 - 10:06 PM
Yes, if everyone follows the best practices there would be no issues. So bad it's not like that :)/>/>
Also, apis are protected against writing, but just of new values, so you can't add new variables to them but you can change one.
And doing
Also, apis are protected against writing, but just of new values, so you can't add new variables to them but you can change one.
And doing
someApiName = someValue
wouldn't make the api dissapear, it would just create a global variable with that value. You can then set it to nil and you get the api back. (Not tested)Posted 31 May 2012 - 10:12 PM
wouldn't make the api dissapear, it would just create a global variable with that value. You can then set it to nil and you get the api back. (Not tested)
Let's face it, though, if you don't verify your values returned from a function, you won't check through the global metatable and make sure there's no variables overriding them, every time you use it :)/>/>