The thing I want to prevent is this:
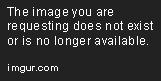
function limitRead( nLength, cReplaceChar )
term.setCursorBlink( true )
nLength = nLength or -1 -- -1 is unlimited
sReturnString = ""
xPos, yPos = term.getCursorPos()
while true do
event, char = os.pullEvent()
if nLength ~= -1 and string.len( sReturnString ) >= nLength then term.setCursorBlink( false ); return sReturnString end -- Length check
if event == "char" then sReturnString = sReturnString .. char
elseif event == "key" and char == 28 then term.setCursorBlink( false ); return sReturnString -- Enter
elseif event == "key" and char == 14 then -- Backspace
term.setCursorPos( xPos, yPos )
term.write( string.rep( " ", string.len( sReturnString ) ) )
sReturnString = string.sub( sReturnString, 1, string.len( sReturnString )-1 )
term.setCursorPos( xPos, yPos )
if not cReplaceChar then term.write( sReturnString )
else term.write( string.rep( cReplaceChar, string.len( sReturnString ) ) ) end
end
term.setCursorPos( xPos, yPos )
term.write( string.rep( " ", string.len( sReturnString ) ) )
term.setCursorPos( xPos, yPos )
if not cReplaceChar then term.write( sReturnString )
else term.write( string.rep( cReplaceChar, string.len( sReturnString ) ) ) end
end
end
I think you actually sent me this a long time ago, but I lost it :D/>/>It's not the most elegant, or efficient for that matter, of a solution, but it works for what you need. I wrote this a long while ago so I can't really provide much support for it.function limitRead( nLength, cReplaceChar ) term.setCursorBlink( true ) nLength = nLength or -1 -- -1 is unlimited sReturnString = "" xPos, yPos = term.getCursorPos() while true do event, char = os.pullEvent() if nLength ~= -1 and string.len( sReturnString ) >= nLength then term.setCursorBlink( false ); return sReturnString end -- Length check if event == "char" then sReturnString = sReturnString .. char elseif event == "key" and char == 28 then term.setCursorBlink( false ); return sReturnString -- Enter elseif event == "key" and char == 14 then -- Backspace term.setCursorPos( xPos, yPos ) term.write( string.rep( " ", string.len( sReturnString ) ) ) sReturnString = string.sub( sReturnString, 1, string.len( sReturnString )-1 ) term.setCursorPos( xPos, yPos ) if not cReplaceChar then term.write( sReturnString ) else term.write( string.rep( cReplaceChar, string.len( sReturnString ) ) ) end end term.setCursorPos( xPos, yPos ) term.write( string.rep( " ", string.len( sReturnString ) ) ) term.setCursorPos( xPos, yPos ) if not cReplaceChar then term.write( sReturnString ) else term.write( string.rep( cReplaceChar, string.len( sReturnString ) ) ) end end end
Sorry for the spacing, but when I post from pastebin to the forums it usually messes the indentation up horribly.
limitRead(14, '*')
for a 14 character password that looks like this when you type it in:
**************
limitRead(-1, '*')