Posted 08 November 2012 - 07:28 AM
Hi!
I am trying to code a program that will show the item count of specific items (such as diamonds, iron, gold, etc) in an easy to read format, that is constantly being updated and shown on a 2 high 4 wide monitor. This is how long I've gotten so far :P/>/> :
this is what the data looks like:
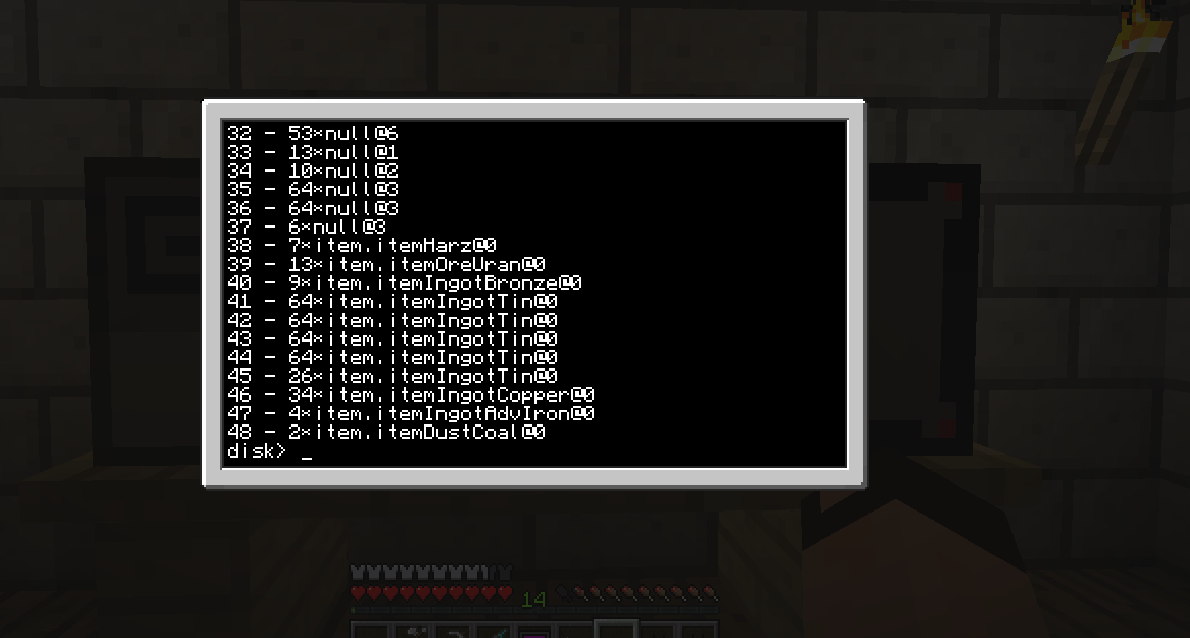
My question(s):
- How do I extract these item names and numbers reliably?
- How can I add, for example, all the iron ingots listed reliably?
Thanks in advance.
I am trying to code a program that will show the item count of specific items (such as diamonds, iron, gold, etc) in an easy to read format, that is constantly being updated and shown on a 2 high 4 wide monitor. This is how long I've gotten so far :P/>/> :
------------- Load API for sensors ------------
os.unloadAPI("sensors")
os.loadAPI("/rom/apis/sensors")
------------- Dictionary Printer --------------
function printDict(data)
for i,v in pairs(data) do
print(tostring(i).." - "..tostring(v))
end
end
------------- TheCode -------------------------
--what side is controller on?
ctrl = sensors.getController()
--list all the sensors and name them
data = sensors.getSensors(ctrl)
invSensorA = data[1]
--list all the probes of specific sensors and name them
data = sensors.getProbes(ctrl,invSensorA)
inventoryContent = data[3]
--list all targets of specific sensor+probe and name them
data = sensors.getAvailableTargetsforProbe(ctrl,invSensorA,inventoryContent)
chestLeftUp = data[6]
data = sensors.getSensorReadingAsDict(ctrl,invSensorA,chestLeftUp,inventoryContent)
printDict(data)
this is what the data looks like:
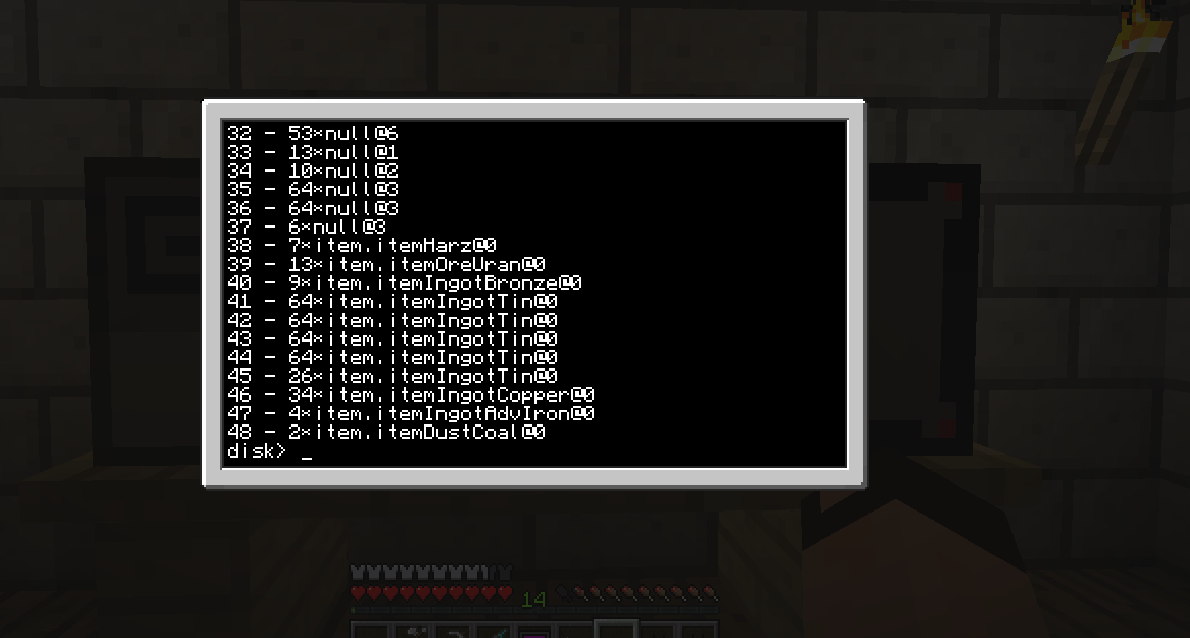
My question(s):
- How do I extract these item names and numbers reliably?
- How can I add, for example, all the iron ingots listed reliably?
Thanks in advance.