Ok, imagine(this is but one example of many different things I want to be able to do.) that I have a house with 6 different floors. I want one computer for each of the six floors that can toggle the lights on/off, via user input. However, I also want a 'mother' computer that can send rednet signals to each of the six computers telling them how to turn the lights on and off. Basically, I want to know how I can make a computer wait for user input AND wait for a rednet signal and act upon them when the get there. Sorry if I have worded this badly, and any help would be greatly appreciated.
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
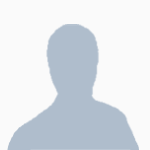
[Question] How can I do this....
Started by deity12, 20 November 2012 - 03:13 PMPosted 20 November 2012 - 04:13 PM
Sorry the title isn't very descriptive but I can't seem to fit what I want to do in it…
Ok, imagine(this is but one example of many different things I want to be able to do.) that I have a house with 6 different floors. I want one computer for each of the six floors that can toggle the lights on/off, via user input. However, I also want a 'mother' computer that can send rednet signals to each of the six computers telling them how to turn the lights on and off. Basically, I want to know how I can make a computer wait for user input AND wait for a rednet signal and act upon them when the get there. Sorry if I have worded this badly, and any help would be greatly appreciated.
Ok, imagine(this is but one example of many different things I want to be able to do.) that I have a house with 6 different floors. I want one computer for each of the six floors that can toggle the lights on/off, via user input. However, I also want a 'mother' computer that can send rednet signals to each of the six computers telling them how to turn the lights on and off. Basically, I want to know how I can make a computer wait for user input AND wait for a rednet signal and act upon them when the get there. Sorry if I have worded this badly, and any help would be greatly appreciated.
Posted 20 November 2012 - 04:19 PM
This is relatively advanced. I would recommend learning Lua a bit more and getting experience with the CC APIs. After that, you'll look back and say, "Pshhh! I know that!" Trust me, I know. :(/>/>
Posted 20 November 2012 - 04:43 PM
Look over the os.pullEvent(), it does what you want.
Posted 22 November 2012 - 05:58 PM
Sorry, for being so terrible, but all I can see is that os.pullEvent wait for me to do something, my question is, how can I wait for two thing to happen at the same time? A code example would be appreciated, as I do find i learn this best from just looking at code, messing around with it untilLook over the os.pullEvent(), it does what you want.
i finally understand it. If it's too and it's way over my head I'll appreciate it anyway.
Posted 22 November 2012 - 06:07 PM
You could use the parallel api. It's somewhat more advanced, but you can give it a go. Here's a sample.
Of course you would change the code inside of those functions to do what you want, but the parallel api will run those two functions at the same time allowing you to both get user input while at the same time receiving rednet messages. The "waitForAny" part just means "wait for any of the given functions to finish and then return true".
rednet.open('top')
function getRednet()
id,message = rednet.receive()
print(message) --Change this to the code you have for changing light status from mother computer
end
function getInput()
input = io.read()
print(input) --Change this to the code you have for changing light status from user input
end
parallel.waitForAny(getRednet, getInput)
Of course you would change the code inside of those functions to do what you want, but the parallel api will run those two functions at the same time allowing you to both get user input while at the same time receiving rednet messages. The "waitForAny" part just means "wait for any of the given functions to finish and then return true".
Posted 23 November 2012 - 03:32 AM
If you call os.pullEvent() without an argument, it will pull any event rather than only a specified type of event. So you do something like
repeat
t_event = {os.pullEvent()}
if t_event[1] == "char" then --do something with the character
elseif t_event[1] == "rednet_message" then --do something with the message
end
until t_event[2] == "X" --an optional input to exit the loop
Posted 23 November 2012 - 03:39 AM
If you call os.pullEvent() without an argument, it will pull any event rather than only a specified type of event. So you do something likerepeat t_event = {os.pullEvent()} if t_event[1] == "char" then --do something with the character elseif t_event[1] == "rednet_message" then --do something with the message end until t_event[2] == "X" --an optional input to exit the loop
Why use a table?
while true do
e, p1, p2, p3 = os.pullEvent()
if e == "char" then
elseif e == "rednet_message" then
end
end
But I guess it makes no different
Posted 23 November 2012 - 05:18 AM
Everything is tables anyway. On the other hand, I avoid "while true do … end" loops whenever possible, because if you can do something else then you almost certainly should.
Posted 23 November 2012 - 05:57 AM
I feel like using the parallel api in combination with os.pullEvent() would be the best way to go, although it is true that you could accomplish this only with os.pullEvent(). With the parallel api however the user can input commands even while the computer is performing other tasks for the mother computer.
Posted 23 November 2012 - 01:36 PM
For more complex string inputs (such as a rednet chat system), the parallel api would be an easier way to handle it. But for a simple task like turning lights on and off (calling an elevator, activating an alarm, anything where you'd just press a button or flip a switch), you probably only need to pull single characters or just key events (or mouse clicks, or even redstone events from nearby buttons/switches).
If you can use single events for inputs, os.pullEvent is much easier and more efficient.
If you can use single events for inputs, os.pullEvent is much easier and more efficient.