This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
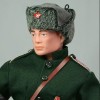
Waiting for text and running a timer simultainiously.
Started by samdeman22, 23 November 2012 - 08:51 AMPosted 23 November 2012 - 09:51 AM
I have a timer going down every second and I want to be able to enter arguments while it's going. Might I have to use 2 computers and redstone ( one computer enters text and the other counts down ) or can the parrallel API help me?
Posted 23 November 2012 - 10:17 AM
Timers operate regardless of your input or any kind of loop. They trigger an event once they expire and return a table as parameter when started and ended. The table returned when the timer is created will match that of the table returned when it ends, therefore allowing you to match timers with certain aspects of your scripts. But, I digress.
It would seem you don't need parallel or the measures that you had explained, but if you want to have timer be checked while your entering input, then you might have to write your own read routine to check for character events and timers. That shouldn't be too difficult, so good luck!
It would seem you don't need parallel or the measures that you had explained, but if you want to have timer be checked while your entering input, then you might have to write your own read routine to check for character events and timers. That shouldn't be too difficult, so good luck!
Posted 23 November 2012 - 10:21 AM
oh, there are built-in lua timers? I made my own in a "while true do" loop. Could you explain the timer to meh?
Posted 23 November 2012 - 10:28 AM
I found it on the cc wiki. I'm familiar with callbacks and things but how does this work? It just says it returns a table with the id of the timer.
Posted 23 November 2012 - 10:28 AM
Sure.
Here is how you would create a timer:
To check if a timer has ended, I would wait and catch it as an event.
Here's how that might look:
Here is how you would create a timer:
local timer = os.startTimer(5)
'5' is the time in seconds that I want the timer to be active for. 'timer' as a variable will be a table.To check if a timer has ended, I would wait and catch it as an event.
Here's how that might look:
local timer = os.startTimer(5)
local event, p1 = os.pullEvent()
if event == "timer" and p1 == timer then
print("Success!")
end
As you can see, p1 is the same timer table as timer when we initialized it. Again, this allows us to match timer events to their specific timers.Posted 23 November 2012 - 10:30 AM
so p1 would be the event I want to call. I'll try it out.
Posted 23 November 2012 - 10:33 AM
No, p1 is the timer table returned when the "timer" event is triggered.so p1 would be the event I want to call. I'll try it out.
Posted 23 November 2012 - 10:36 AM
ah, another problem what if I want to save the current time on the timer to a file? (incase the computer is turned off), or preferably, how do I prevent the user from doing "Ctrl+S" or "Ctrl+t" etc.?
Posted 23 November 2012 - 10:48 AM
To save the current time in a timer, you would have to have your own timer object that keeps track of the time. Unfortunately, the default timers within CC do not support this feature as they are hardcoded objects within the mod.
To prevent program termination use the following as header to your script:
As for shutting down the computer, that part is hardcoded and therefore cannot be prevented from within ComputerCraft.
To prevent program termination use the following as header to your script:
os.pullEvent = os.pullEventRaw
This makes every reference that any function called within your program makes to os.pullEvent a call to os.pullEventRaw which does not support termination events.As for shutting down the computer, that part is hardcoded and therefore cannot be prevented from within ComputerCraft.
Posted 23 November 2012 - 10:49 AM
You cant prevent from Ctrl+S and Ctrl+R, but with:
os.pullEvent=os.pullEventRaw
You can prevent Ctrl+TPosted 23 November 2012 - 11:00 AM
alright then, and adding more parts to the event ( local event p1, p2, p3 = os.pullEventRaw() ) would mean I can do
if event == "timer" and p1 == timer then
do stuff
elseif event == "timer" and p2 == timer then
do different stuff
elseif event == "timer" and p3 == timer then
do even more different stuff
end
Posted 23 November 2012 - 11:24 AM
alright then, and adding more parts to the event ( local event p1, p2, p3 = os.pullEventRaw() ) would mean I can doif event == "timer" and p1 == timer then do stuff elseif event == "timer" and p2 == timer then do different stuff elseif event == "timer" and p3 == timer then do even more different stuff end
Not exactly; only one timer will trigger an event.
Only one parameter is returned by the timer event, that being p1 (the unique timer id).
You could have several timers and check them against p1 to execute the right set of instrouctions.
Posted 01 December 2012 - 06:47 AM
a new problem arises: about loops within loops
so will the "while reset ~= true" loop stop as soon as reset = anything else? or will I have to break the conditional loop to satisfy it?
while true do
while reset ~= true do
-- stuff in here
while true do
if blah blah then
reset = true
break
elseif blah blooh then
reset = true
break
end
end -- the conditional loop
end -- the ?reset loop
end -- the program
so will the "while reset ~= true" loop stop as soon as reset = anything else? or will I have to break the conditional loop to satisfy it?
Posted 01 December 2012 - 07:09 AM
Well, I can reveal what I'm making now. I'm making the computer from LOST, It will be in 3 parts: the cpu; counts down and sends redstone signals between the terminal and the monitorPC, the terminal; accepts input and isnt hard at all and finally the monitorPC; this is the most difficult since I can't do anything grpahical and I have no idea where to start on colors and stuff.
What I'm trying to say is can someone help me make the code for the monitor, I want it to be a 2 monitor rig with the text fairly big, as well as it doing the countdown I want it to do the hyrogliphs when the countdown reaches 0. Google/youtube it if you dont know what it looks like.
Thanksall
What I'm trying to say is can someone help me make the code for the monitor, I want it to be a 2 monitor rig with the text fairly big, as well as it doing the countdown I want it to do the hyrogliphs when the countdown reaches 0. Google/youtube it if you dont know what it looks like.
Thanksall
Posted 01 December 2012 - 01:05 PM
Big text:What I'm trying to say is can someone help me make the code for the monitor, I want it to be a 2 monitor rig with the text fairly big, as well as it doing the countdown I want it to do the hyrogliphs when the countdown reaches 0. Google/youtube it if you dont know what it looks like.
mon = peripheral.wrap(the_side_of_it)
mon.setTextScale(5)
mon.write(time_left)
For the hyroglyphs, there aren't any heryglyph in CC hat I am aware, but you could just write random stuff in the screen