This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
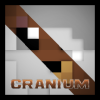
Detecting advanced computers
Started by Cranium, 05 December 2012 - 02:58 AMPosted 05 December 2012 - 03:58 AM
I know now that since we have advanced computers, if you try to use an advanced program on a normal computer, you will get an error. Is it possible to detect that, just like detecting the http enabled before running an errored code? I want to be able to make a program that can be used by both advanced computers and regular computers. I took a look at some of the new files available in the mod, but didn't see anything like I did with the http api error.(if http then)
Posted 05 December 2012 - 04:22 AM
term.isColor()
Posted 05 December 2012 - 04:38 AM
Lemme guess….. This is in the wiki -_-/>term.isColor()
Posted 05 December 2012 - 04:51 AM
I think you might want something like below, but it would be lengthy for a program, because you'd have to have the code twice.
If you were talking about code to just ignore the color commands, I have been wondering about the same thing :P/>. I was wondering if there was something like this in Lua:
if term.isColor() then
term.setTextColor(colors.lime)
print("Hello!")
term.setTextColor(colors.white)
else
print("Hello!")
end
If you were talking about code to just ignore the color commands, I have been wondering about the same thing :P/>. I was wondering if there was something like this in Lua:
if not term.isColor() then
string.ignore("term.setTextColor()")
string.ignore("term.setBackgroundColor()")
end
Posted 05 December 2012 - 04:53 AM
If you were talking about code to just ignore the color commands, I have been wondering about the same thing :P/>. I was wondering if there was something like this in Lua:if not term.isColor() then string.ignore("term.setTextColor()") string.ignore("term.setBackgroundColor()") end
I wish it were that easy….
Posted 05 December 2012 - 04:55 AM
well, make your own custom file running program…
EDIT: I got it! make a program that will run through your file and put – infront of any unsupported command.
EDIT: I got it! make a program that will run through your file and put – infront of any unsupported command.
Posted 05 December 2012 - 04:57 AM
in my battleship game I'm working on (basically finished, just needs some clean-up), I did this…
Then I just called doColor to set the color instead of calling the term functions directly.
:edit: :facepalm: How am I just NOW discovering that basic computers can do black text on white backgrounds? Never seen it done and never thought to test it myself until just now. So battleship needs more work after all, can greatly improve the rendering of the basic computer version with this knowledge!
local function doColor(fg,bg)
end
if term.isColor() then
doColor=function(fg,bg)
if fg then term.setForegroundColor(fg) end
if bg then term.setBackgroundColor(bg) end
end
end
Then I just called doColor to set the color instead of calling the term functions directly.
:edit: :facepalm: How am I just NOW discovering that basic computers can do black text on white backgrounds? Never seen it done and never thought to test it myself until just now. So battleship needs more work after all, can greatly improve the rendering of the basic computer version with this knowledge!
Posted 05 December 2012 - 04:58 AM
If you were talking about code to just ignore the color commands, I have been wondering about the same thing :P/>. I was wondering if there was something like this in Lua:if not term.isColor() then string.ignore("term.setTextColor()") string.ignore("term.setBackgroundColor()") end
No, not even a little bit.
Also, if you just want color to show up on advanced machines for what you're printing, a simple ternary operation will do nicely.
term.setTextColor(term.isColor() and colors.white or colors.black)
term.setBackgroundColor(term.isColor() and colors.blue or colors.white)
term.write("Some Highlighted Text")
term.setTextColor(colors.white)
term.setBackgroundColor(colors.black)
Posted 05 December 2012 - 05:40 AM
Nice use of the OR operation.No, not even a little bit.
Also, if you just want color to show up on advanced machines for what you're printing, a simple ternary operation will do nicely.term.setTextColor(term.isColor() and colors.white or colors.black) term.setBackgroundColor(term.isColor() and colors.blue or colors.white) term.write("Some Highlighted Text") term.setTextColor(colors.white) term.setBackgroundColor(colors.black)
To explain how this works in a non color computer you can use term.setTextColor() and term.setBackgroundColor() you can only use black or white . If you give those functions any other input it will fail and give an error. The code from Lyqyd works because if it is color it returns the color if not it returns the other color the function then sets the color.
another way to do it is
local backColor = colors.red
local textColor = colors.blue
if term.isColor() then
backColor = colors.white
textColor = colors.black
end
term.setBackgroundColor(backColor)
term.setTextColor(textColor)
print("hello")
Posted 05 December 2012 - 05:53 AM
Actually, I think the term.setBackgroundColor(term.isColor() and colors.red or colors.black) would be exactly what I need. I think I'll have to update SmartPaste with that.
Quick question though, from what I got from this is that colors.black and colors.white can be used on non-colored computers? Or should I just use the term.setBackgroundColor(term.isColor() and colors.red) only?
(only reason i ask these questions is because i can't test at work :/)
Quick question though, from what I got from this is that colors.black and colors.white can be used on non-colored computers? Or should I just use the term.setBackgroundColor(term.isColor() and colors.red) only?
(only reason i ask these questions is because i can't test at work :/)
Posted 05 December 2012 - 06:34 AM
Yep, non-color computers can use any combination of black and white, but white-on-white is less than useful.
Posted 05 December 2012 - 07:24 AM
I wish it were that easy….
Actually, it is that easy. I do this for almost all of my adv. programs - overwriting the term functions.
local setTextColor = term.setTextColor
local setBackgroundColor = term.setBackgroundColor
function term.setTextColor(...)
if term.isColor() then
setTextColor(...)
end
end
function term.setBackgroundColor(...)
if term.isColor() then
setTextColor(...)
end
end
Posted 05 December 2012 - 08:45 AM
I wish it were that easy….
Actually, it is that easy. I do this for almost all of my adv. programs - overwriting the term functions.local setTextColor = term.setTextColor local setBackgroundColor = term.setBackgroundColor function term.setTextColor(...) if term.isColor() then setTextColor(...) end end function term.setBackgroundColor(...) if term.isColor() then setTextColor(...) end end
Unfortunately, this would remove entirely the ability to set the color on non-advanced computers, rather than just limiting them to the colors they can use.
Posted 05 December 2012 - 10:06 AM
Doesn't matter to me since I don't use background colors other than for screwing around, and having black on a black background wouldn't make much sense :lol:/>
But at that point, with my method, you may as well just make your own local functions to do it.
But at that point, with my method, you may as well just make your own local functions to do it.
local allowed = {[1]=true, [32768]=true}
function setFG(color)
if not term.isColor() and not allowed[color] then
return
end
term.setTextColor(color)
end
function setBG(color)
if not term.isColor() and not allowed[color] then
return
end
term.setBackgroundColor(color)
end