x=0
while turtle.detectDown() do
turtle.forward()
turtle.digUp()
turtle.dig()
turtle.select(2)
turtle.placeDown()
turtle.select (1)
x=x+2
print (""..x.."")
if turtle.getFuelLevel() < 1 then
do turtle.select(1)
turtle.refuel(1)
end
else
end
end
So far it works a treat but there is some things I want it to do that I can't seem to figure out. The first thing would be the ability to count the blocks picked up rather than adding 2 to x every time it hits that part of the script. The second is the ability to stop the script without having to reload MineCraft (currently it runs for ever but when it runs out of fuel it just sits there, still running the script.) Third is being able to detect when it's inventory is full then going back to where it started to put items in a chest. I'm sorry if it seems like a big request. Thanks in advance.
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
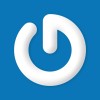
[Question] Mining program features.
Started by ShinyJijo, 16 December 2012 - 12:47 AMPosted 16 December 2012 - 01:47 AM
Ok guys. I have spent the last few hours creating my fantastic first program for my turtle. What I am trying to do is create a program that tunnels a 2x1 tunnel. What I have so far:
Posted 16 December 2012 - 02:03 AM
ok lets tackle this one bit at a time.
first. counting blocks do this.
making sense so far?
first. counting blocks do this.
dugBlocks = 0
function dig()
while turtle.detectUp() do
if turtle.digUp() then
dugBlocks = dugBlocks + 1
sleep(0.8) -- let sand and gravel fall
end
end
while turtle.detect() do
if turtle.dig() then
dugBlocks = dugBlocks + 1
sleep(0.8) -- let sand and gravel fall
end
end
function fillBelow()
while not turtle.detectDown() do -- just incase slot 1 is gravel and the hole below is large
if turtle.getItemCount(2) > 0 then
turtle.select(2)
turtle.placeDown()
else
break -- if the second slot is empty it ignores placing down
end
end
end
function checkFuel()
if turtle.getFuelLevel() < 1 then
turtle.select(1)
turtle.refuel()
end
end
while true do
turtle.forward()
dig()
fillBelow()
checkFuel()
end
making sense so far?
Posted 16 December 2012 - 02:10 AM
That makes sense. The only thing I don't get is the while true do at the bottom there. Does that just make the functions loop?
Posted 16 December 2012 - 02:12 AM
yes it does, it makes it an infinite loop, unless the program is terminated or errors.
oops. i should mention put a sleep(0.1) before that last end or it will error out.
with your while not turtle.detectDown() do meant that it would only run when there was no block below it, and when there was it would stop.
oops. i should mention put a sleep(0.1) before that last end or it will error out.
with your while not turtle.detectDown() do meant that it would only run when there was no block below it, and when there was it would stop.
Posted 16 December 2012 - 02:17 AM
ok so next bit. terminating the program is as easy as holding control + t. this will terminate the program. however if you wish to do something like a key binding change the infinite loop to this
For ComputerCraft 1.4
For ComputerCraft 1.3
For ComputerCraft 1.4
Spoiler
timer = os.startTimer(0.1)
while true do
event, param = os.pullEvent()
if event == "key" and param == keys.s then -- replace s with any key you want except escape :)/>
elseif event == "timer" then
turtle.forward()
dig()
fillBelow()
checkFuel()
end
end
For ComputerCraft 1.3
Spoiler
use the code from 1.4 except where there is keys.s replace it with a number from here http://www.minecraftwiki.net/wiki/Key_codesPosted 16 December 2012 - 02:46 AM
Okay. I was trying out the end script keybind at the end there but I think I will just stick with Ctrl+T. Also with that while not turtle.detectDown() problem, would that be solvable by merging it into the dig() function instead of being its own function?
EDIT: Apparently not…
EDIT: Apparently not…
Posted 16 December 2012 - 02:48 AM
the fillBelow() fills the ground below. is this not why u were using turtle.detectDown() ?
Posted 16 December 2012 - 02:49 AM
ok now for the third problem.
Inventory detection and drop off. firstly, would you want it after dropping off its items going back to where it was an continuing?
Inventory detection and drop off. firstly, would you want it after dropping off its items going back to where it was an continuing?
Posted 16 December 2012 - 02:58 AM
Yes, i was using turtle.detectDown() to place blocks underneath it in case it ran into a cave or ravine.the fillBelow() fills the ground below. is this not why u were using turtle.detectDown() ?
I would like it dropping off its items then returning to where it was.ok now for the third problem.
Inventory detection and drop off. firstly, would you want it after dropping off its items going back to where it was an continuing?
Posted 16 December 2012 - 03:00 AM
Yes, i was using turtle.detectDown() to place blocks underneath it in case it ran into a cave or ravine.
Well in that case the fillBelow() will do that for you, it also has gravel or sand support like dig if you didn't notice :)/>
I would like it dropping off its items then returning to where it was.
ok would you be adverse to using an enderchest? that is the quickest and nicest :)/>
Posted 16 December 2012 - 03:17 AM
But didn't you say that turtle.detectDown() would stop the script? I tested it and also found out that when it went over a block it stopped moving. About the enderchest, i think that is a good idea.Yes, i was using turtle.detectDown() to place blocks underneath it in case it ran into a cave or ravine.
Well in that case the fillBelow() will do that for you, it also has gravel or sand support like dig if you didn't notice :)/>I would like it dropping off its items then returning to where it was.
ok would you be adverse to using an enderchest? that is the quickest and nicest :)/>
Posted 16 December 2012 - 03:36 AM
I pauses with my version? odd. I'll check that in a minute.
Ok so here is the entire code again, this time with drop off:
http://pastebin.com/zqJvHtHM
about to test but should work :)/>
Ok so here is the entire code again, this time with drop off:
http://pastebin.com/zqJvHtHM
about to test but should work :)/>
Posted 16 December 2012 - 03:45 AM
I was reading the code and maxItemsDropFromBLock should equal 8. The minecraft wiki said so.
Posted 16 December 2012 - 03:48 AM
Ahh ok. I honestly couldn't remember I was tossing up between 4 and 8. lol, damn. other than that, how does it look?
Posted 16 December 2012 - 03:51 AM
Either i am blind but you haven't employed use of the functions like turnAround etc. or do the functions still get executed even though they aren't in the while true do loop?
Posted 16 December 2012 - 03:54 AM
the turnAround() is used in dropOffItems() which is used in checInvSpace() which is called each time before digging
Posted 16 December 2012 - 04:09 AM
Thanks for your help. just some things i need to clear up before i go to bed here.
at this pont:
at this pont:
for i = 1, 16 do
if i ~= enderchestSlot then
while not turtle.getItemCount() == 0 do
and this point:
for i = 1, 16 do
turtle.select(i)
if turtle.compare() and turtle.getItemSpace(i) > maxItemDropFromBlock then
what does i mean?Posted 16 December 2012 - 04:13 AM
Thanks for your help. just some things i need to clear up before i go to bed here.
at this pont:and this point:for i = 1, 16 do if i ~= enderchestSlot then while not turtle.getItemCount() == 0 do
what does i mean?for i = 1, 16 do turtle.select(i) if turtle.compare() and turtle.getItemSpace(i) > maxItemDropFromBlock then
ok so i is actually a variable. so what we are doing in these for loops is declaring a new variable called i and assigning it the number 1.
now please do note that normal naming convention says that you would give your variables meaningful names, however they make they exception for 1 letter variables when for loops are being used. but i can be anything, it could read
for slot = 1, 16 do
if you wish to do that. :)/>
hope that was clear enough to understand :)/>
Posted 16 December 2012 - 04:16 AM
so does writing i = 1,16 assign the numbers 1-16 to i? other than that i get it.Thanks for your help. just some things i need to clear up before i go to bed here.
at this pont:and this point:for i = 1, 16 do if i ~= enderchestSlot then while not turtle.getItemCount() == 0 do
what does i mean?for i = 1, 16 do turtle.select(i) if turtle.compare() and turtle.getItemSpace(i) > maxItemDropFromBlock then
ok so i is actually a variable. so what we are doing in these for loops is declaring a new variable called i and assigning it the number 1.
now please do note that normal naming convention says that you would give your variables meaningful names, however they make they exception for 1 letter variables when for loops are being used. but i can be anything, it could readfor slot = 1, 16 do
if you wish to do that. :)/>
hope that was clear enough to understand :)/>
Posted 16 December 2012 - 04:19 AM
so does writing i = 1,16 assign the numbers 1-16 to i? other than that i get it.Thanks for your help. just some things i need to clear up before i go to bed here.
at this pont:and this point:for i = 1, 16 do if i ~= enderchestSlot then while not turtle.getItemCount() == 0 do
what does i mean?for i = 1, 16 do turtle.select(i) if turtle.compare() and turtle.getItemSpace(i) > maxItemDropFromBlock then
ok so i is actually a variable. so what we are doing in these for loops is declaring a new variable called i and assigning it the number 1.
now please do note that normal naming convention says that you would give your variables meaningful names, however they make they exception for 1 letter variables when for loops are being used. but i can be anything, it could readfor slot = 1, 16 do
if you wish to do that. :)/>
hope that was clear enough to understand :)/>
it starts it off at 1, and then each time it loops back to that point it increments it by one, until it gets to 16.
if it was for i = 1, 16, 2 do then it would do the same thing but increment by 2 each time
make sense?
Posted 16 December 2012 - 04:21 AM
I see now. And you use that to choose the right slot when placing the enderchest?so does writing i = 1,16 assign the numbers 1-16 to i? other than that i get it.Thanks for your help. just some things i need to clear up before i go to bed here.
at this pont:and this point:for i = 1, 16 do if i ~= enderchestSlot then while not turtle.getItemCount() == 0 do
what does i mean?for i = 1, 16 do turtle.select(i) if turtle.compare() and turtle.getItemSpace(i) > maxItemDropFromBlock then
ok so i is actually a variable. so what we are doing in these for loops is declaring a new variable called i and assigning it the number 1.
now please do note that normal naming convention says that you would give your variables meaningful names, however they make they exception for 1 letter variables when for loops are being used. but i can be anything, it could readfor slot = 1, 16 do
if you wish to do that. :)/>
hope that was clear enough to understand :)/>
it starts it off at 1, and then each time it loops back to that point it increments it by one, until it gets to 16.
if it was for i = 1, 16, 2 do then it would do the same thing but increment by 2 each time
make sense?
Posted 16 December 2012 - 04:27 AM
I see now. And you use that to choose the right slot when placing the enderchest?
the if i ~= enderchestSlot means if i is not the enderchest slot ~= means not equal to. I guess there is no real reason to have it there since its dumping into that chest.
also i suggest that you put the following piece of code in before the infinited loop
-- other code here
if turtle.getItemCount(enderchestSlot) < 1 then
collectChest()
turnAround()
end
-- infinite loop here
I suggest adding this so that if the turtle is mid drop off into the chest when the SMP or SSP closes when it starts it will collect the chest, turn around, and keep working :)/>
Posted 16 December 2012 - 04:29 AM
Thanks for the help friend. I have got to go now cya :)/>I see now. And you use that to choose the right slot when placing the enderchest?
the if i ~= enderchestSlot means if i is not the enderchest slot ~= means not equal to. I guess there is no real reason to have it there since its dumping into that chest.
also i suggest that you put the following piece of code in before the infinited loop-- other code here if turtle.getItemCount(enderchestSlot) < 1 then collectChest() turnAround() end -- infinite loop here
I suggest adding this so that if the turtle is mid drop off into the chest when the SMP or SSP closes when it starts it will collect the chest, turn around, and keep working :)/>
Posted 16 December 2012 - 04:31 AM
your welcome :)/>