Hello, I have started picking up turtle programming lately as I have programmed java/c++ for a few years now, and have learned fairly quickly.
I have been trying to create a quarry program, but I cannot figure out where it is going wrong. The program is supposed to dig forward X blocks, turn right/left, move forward, then turn right/left again, then repeating the whole process. It runs the first section fine, but it is when it turns around that something goes wrong, moving forward an extra space before needing to turn around again. I have tried printing out numbers of the for loop to figure out if it was starting under a different value, but to no success.
By the way, I know that as of now the program will not start digging down properly. I am trying to get one layer perfect before I worry about the rest.
Also, if someone could clarify how remainders (X%2=0 for example) work with turtles that would be awesome. You can see where it would come in handy with my side=0, side=2, side=4, etc.
Pictures of the problem:
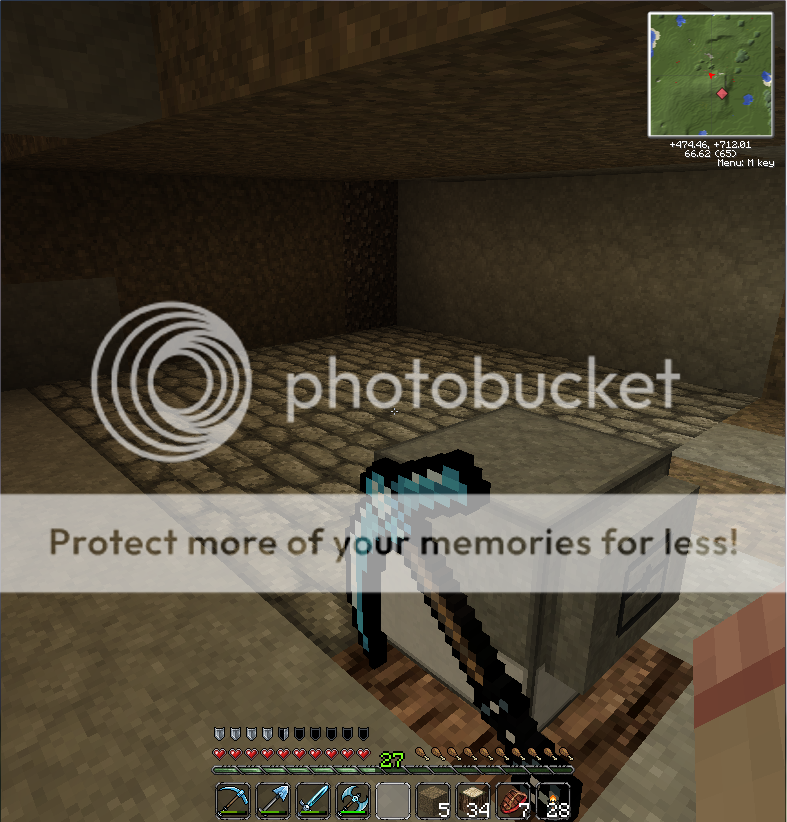
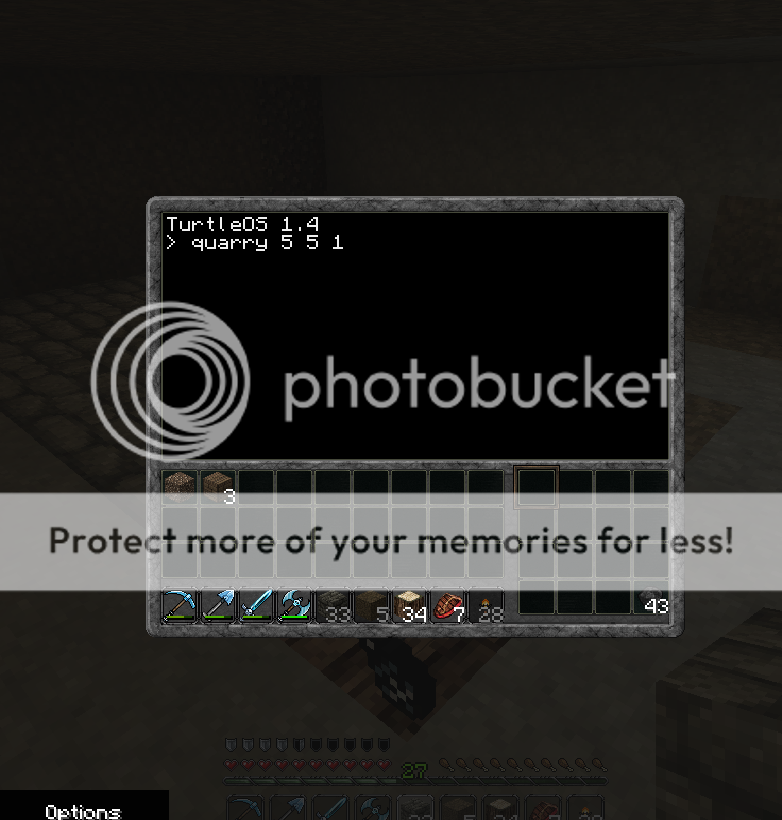
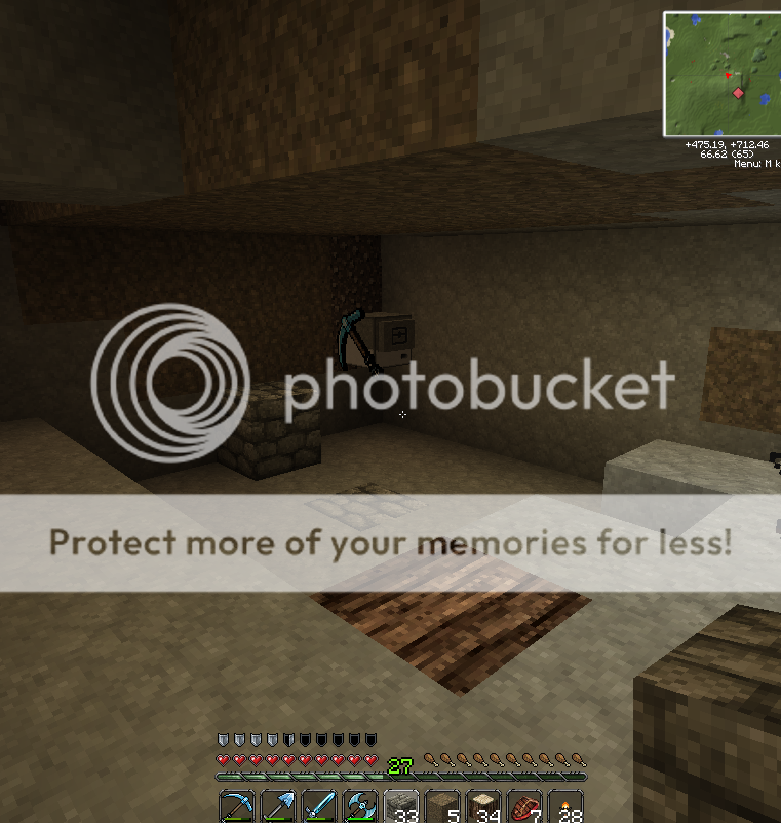
Program code:
local tArgs = { ... }
local length = tonumber(tArgs[1])
local width = tonumber(tArgs[2])
local depth = tonumber(tArgs[3])
local down=0
local side=0
-- Functions --
function fuel()
if turtle.getFuelLevel() < 5 then
turtle.select(16)
turtle.refuel(1)
turtle.select(1)
end
end
-- Program --
turtle.forward()
fuel()
while down<=depth do
repeat
repeat
for i=1, length do
turtle.digDown()
turtle.forward()
write(i)
end
i=0
fuel()
if side==0 or side==2 or side==4 or side==6 or side==8 or side==10 then
turtle.turnRight()
turtle.forward()
turtle.turnRight()
else
turtle.turnLeft()
turtle.forward()
turtle.turnLeft()
end
side=side+1
until side==length
side=0
down=down+1
until down==depth
end