Thanks
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
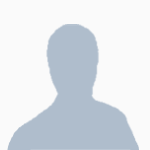
Rednet Confirmation When Sent
Started by ILoveZerg, 20 December 2012 - 02:55 AMPosted 20 December 2012 - 03:55 AM
I am trying to have a system where a computer can send a rednet message and will continue to send the message until it recieves a confimation message back. I cannot seem to thnk of a way to do this. Any help would be appreciated.
Thanks
Thanks
Posted 20 December 2012 - 04:03 AM
You could probably just have a loop, then break out of it when the signal is valid.
This script basically sends a signal to whatever ID, then waits 5 seconds for a response. If it gets a response, it gets out of the loop. Otherwise, it just goes back to the beginning and try again.
EDIT: Just realized you could do this:
But do whatever works for you.
local id, msg
while true do
rednet.send(someID, someSignal)
id, msg = rednet.receive(5)
if id and msg then break end
end
-- do stuff
This script basically sends a signal to whatever ID, then waits 5 seconds for a response. If it gets a response, it gets out of the loop. Otherwise, it just goes back to the beginning and try again.
EDIT: Just realized you could do this:
local id, msg
while not id and not msg do
rednet.send(someID, someSignal)
id, msg = rednet.receive(5)
end
-- do stuff
But do whatever works for you.
Posted 20 December 2012 - 12:35 PM
I would probably do something that more replicates networking in real life by doing the following:
Hope this helps :)/>
- Send out message
- Wait for an "ACK" to be sent back from that same computer (this means all computers need to be setup to send an "ACK" back after receiving any message except an "ACK", and making it wait for an "ACK" from the computer it sent to, means it wont bug out on broadcasts or other terminals sending an "ACK")
- If no "ACK" in say 1 second (should theoretically be enough time, if not bump it up :)/> ) send message again until ACK received.
Hope this helps :)/>
Posted 21 December 2012 - 06:24 AM
One liiiiine~You could just do what Kingdaro said, however the one downside to this is if you are on a server, someone might send out a dummy message and could screw up your entire system since all terminals listen for a message. adding checking for a specific computer ID and message is the way to go if that concerns you. :)/>/>
local id, msg
while true do
rednet.send(someID, someSignal)
id, msg = rednet.receive(5)
if id == someID then break end
end
Posted 21 December 2012 - 07:28 AM
That's…not one line. Or even one liiiiine~.
And for the love of Cloudy's pet peeves, use:
Oh wait, I just realized that it was a one line modification of your previous code.
And for the love of Cloudy's pet peeves, use:
local id, msg
repeat
rednet.send(someID, someSignal)
id, msg = rednet.receive(5)
until id == someID
It is also a pet peeve of mine as well.Oh wait, I just realized that it was a one line modification of your previous code.
Edited on 21 December 2012 - 06:30 AM
Posted 21 December 2012 - 11:54 AM
One liiiiine~You could just do what Kingdaro said, however the one downside to this is if you are on a server, someone might send out a dummy message and could screw up your entire system since all terminals listen for a message. adding checking for a specific computer ID and message is the way to go if that concerns you. :)/>/>local id, msg while true do rednet.send(someID, someSignal) id, msg = rednet.receive(5) if id == someID then break end end
And again what if he doesn't want to program all the computer IDs into the program.
Posted 21 December 2012 - 01:27 PM
Yeah, this makes more logical sense, but eh. Either one works.That's…not one line. Or even one liiiiine~.
And for the love of Cloudy's pet peeves, use:It is also a pet peeve of mine as well.local id, msg repeat rednet.send(someID, someSignal) id, msg = rednet.receive(5) until id == someID
What, like command line arguments?And again what if he doesn't want to program all the computer IDs into the program.
local id, msg
while true do
rednet.send(tonumber(({...})[1]), someSignal)
id, msg = rednet.receive(5)
if id == someID then break end
end
Posted 21 December 2012 - 01:40 PM
What, like command line arguments?
Nah I meant more like one of the systems I used to run. I was adding a new computer every day or so and I didn't then want to go back around to all the computers just to add that new computers ID to a list so they could talk to it, so i made the code dynamic so that all i do is tell the new one what it is and then it broadcasts to the rest what it is, and then they all add it into their table and the save file so they can communicate later.
Posted 21 December 2012 - 01:47 PM
So it's sort of like, you have one computer, with a secret rednet "password" that you send in order to have that computer be able to send and receive signals?
Posted 21 December 2012 - 01:53 PM
yeh basically.
Posted 21 December 2012 - 02:04 PM
You could also make it so that the computer had to be in a specific area, as confirmed by the distance to a few other computers.
But that's doesn't change the functionality of the part that uses the ID's, it just changes how they get into the program.
But that's doesn't change the functionality of the part that uses the ID's, it just changes how they get into the program.