Thank you.
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
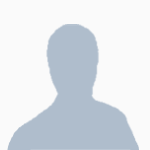
Add a set number of times to loop
Started by Early_Sep_99_Baby, 21 December 2012 - 09:34 AMPosted 21 December 2012 - 10:34 AM
I probably sound like such a noob, but is there a way to have a loop be repeated a set number of times. I know I could just type it out a bunch of times, but i want it to repeat the code 5 times, and honestly I don't have the patience to write out my code again and again. If there is any solution to my problem it would be greatly appreciated.
Thank you.
Thank you.
Posted 21 December 2012 - 10:36 AM
this belongs in ask a pro
use the for command:
use the for command:
for var=1,5 do
print(var)
end
that will output:
1
2
3
4
5
Posted 21 December 2012 - 07:32 PM
You can use something like a repeat loop, or even a while loop.
Repeat loop:
While loop:
Both of those loops should work, and only loop 8 times. Hope I helped!
Repeat loop:
i = 8
repeat
-- code
i = i - 1
until i < 1
While loop:
i = 8
while not i == 0 do
-- code
i = i - 1
end
Both of those loops should work, and only loop 8 times. Hope I helped!
Posted 21 December 2012 - 07:38 PM
you could do
while I = 1, 1 do
-- then the loop
end
Posted 21 December 2012 - 09:06 PM
I suppose you mean:you could dowhile I = 1, 1 do -- then the loop end
for I = 1, 1 do
-- then the loop
end
Which is the same as what PixelToast suggested.Posted 21 December 2012 - 11:06 PM
To OP: The easiest would be to use the for loop, you can also go down in the loop and jump a specific times:
Decrementing:
Incrementing:
Decrementing:
for i = 15, 1, -1 do -- The -1 makes the loop decrement by -1 each step
print(i)
end
Output:15, 14, 13, 12, ..., 3, 2, 1
Incrementing:
for i = 1, 20, 4 do -- The 4 makes it jump 4 each time
print(i)
end
Output:1, 5, 9, 13, 17
Posted 22 December 2012 - 12:07 AM
I almost never use anything other than the standard increment behavior, if I want a multiple of the number of times the loop has actually executed I use i*m (m being my multiple and i being the for index/iteration/whatever). I do very occasionally use a decrement loop, those are pretty useful.
In other words, do what PixelToast says (only use for i=1,5 do, "i" is kinda a standard iteration identifier).
In other words, do what PixelToast says (only use for i=1,5 do, "i" is kinda a standard iteration identifier).
Posted 22 December 2012 - 03:47 AM
i usually use l1 and increase whenever i use a loop inside a loop
Posted 22 December 2012 - 12:28 PM
Yes, technically you can nest a loop and still use "i" as the identifier for the iterator value, but this prevents you from having access to the iterator values for the outer loops. And generally, if you're using nested loops, you'll want to be able to grab those. At least I pretty much always do.