This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
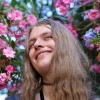
[Lua][Question]How to convert "0.0" into "0"?
Started by Frederikam, 29 December 2012 - 03:41 AMPosted 29 December 2012 - 04:41 AM
This question is simple, I want to know how to convert for example "6.0" into "6". What happens is that when I try to save an int it adds for example ".0" to the int.
Posted 29 December 2012 - 04:45 AM
str = string.match(tostring(myNumber), "(%-?%d+)%.")
See string manipulation and its subsection on patterns in the Lua reference manual.
Posted 29 December 2012 - 04:46 AM
When I tried setting a variable to 6.0, then printed the variable, it returned 6. So I don't see your problem here, lua does this automatically for numbers.This question is simple, I want to know how to convert for example "6.0" into "6". What happens is that when I try to save an int it adds for example ".0" to the int.
Unless you're trying to do this with a string, which Lyqyd has show how to do such a thing.
Posted 29 December 2012 - 04:49 AM
When I tried setting a variable to 6.0, then printed the variable, it returned 6. So I don't see your problem here, lua does this automatically for numbers.This question is simple, I want to know how to convert for example "6.0" into "6". What happens is that when I try to save an int it adds for example ".0" to the int.
Unless you're trying to do this with a string, which Lyqyd has show how to do such a thing.
But I get my "if" and "elseif" to be false because of this.
Posted 29 December 2012 - 04:54 AM
str = string.match(tostring(myNumber), "(%-?%d+)%.")
See string manipulation and its subsection on patterns in the Lua reference manual.
I looked at it, but i do not quite understand any of it, or even know what I should be looking after. I am a newbie to Lua.
Posted 29 December 2012 - 04:54 AM
Let's see the code, please. I think a simpler solution is possible.
Posted 29 December 2012 - 04:56 AM
Let's see the code, please. I think a simpler solution is possible.
curFacing = 0
curX = 0
curY = 0
curZ = 0
curFacingString = UNKNOWN
function fell()
if turtle.dig() then
turtle.dig()
turtle.forward()
turtle.select(2)
turtle.digUp()
turtle.up()
while turtle.compareUp() == true and turtle.digUp() do
turtle.digUp()
turtle.up()
end
while turtle.down() == true do
if turtle.getFuelLevel() == 0 then
turtle.select(1)
turtle.refuel(1)
turtle.select(2)
end
turtle.down()
end
print("Finished tree!")
end
end
function forward()
turtle.forward()
if curFacing == 0 then
curZ = curZ + 1
elseif curFacing == 1 then
curX = CurX - 1
elseif curFacing == 2 then
curZ = curZ - 1
else
curX = curX + 1
end
saveData()
end
function left()
turtle.turnLeft()
if curFacing == 0 then
curFacing = 3
else
curFacing = curFacing - 1
end
saveData()
end
function right()
turtle.turnRight()
if curFacing == 3 then
curFacing = 0
else
curFacing = curFacing + 1
end
saveData()
end
function up()
turtle.up()
curY = curY + 1
saveData()
end
function down()
turtle.down()
curY = curY - 1
saveData()
end
function saveData()
print("saving!")
local file = fs.open("/data", "w")
file.writeLine(curX)
file.writeLine(curY)
file.writeLine(curZ)
file.writeLine(curFacing)
file.close()
end
function loadData()
local file = fs.open("/data", "r")
curX = file.readLine()
curY = file.readLine()
curZ = file.readLine()
curFacing = file.readLine()
print("Save data loaded!")
if curFacing == 0 then
curFacingString = south
elseif curFacing == 1 then
curFacingString = west
elseif curFacing == 2 then
curFacingString = south
elseif curFacing == 3 then
curFacingString = east
else
print("Facing direction unknown!")
end
--print("I am at X="..curX..", Y="..curY..", Z="..curZ.."and I am facing "..curFacingString..".")
end
-- Main function
if fs.exists("/data") then
loadData()
else
print("No data found")
end
print(curX)
print(curY)
print(curZ)
print(curFacing)
if turtle.getFuelLevel
Posted 29 December 2012 - 05:02 AM
You need to tonumber() the values when you load them from the file. Here is an example line to show you what I mean:
curX = tonumber(file.readLine())
They are loaded as strings from the file, so to use them correctly as numbers, you must use tonumber() when loading them.
curX = tonumber(file.readLine())
They are loaded as strings from the file, so to use them correctly as numbers, you must use tonumber() when loading them.
Posted 29 December 2012 - 05:06 AM
You need to tonumber() the values when you load them from the file. Here is an example line to show you what I mean:
curX = tonumber(file.readLine())
They are loaded as strings from the file, so to use them correctly as numbers, you must use tonumber() when loading them.
Okay thanks, I knew that "0.0" can not be an int.
Edit:
I still get a problem.
local file = fs.open("/data", "r")
curX = tonumber(file.readLine())
curY = tonumber(file.readLine())
curZ = tonumber(file.readLine())
curFacing = tonumber(file.readLine())
print("Save data loaded!")
if curFacing == 0 then
curFacingString = south
elseif curFacing == 1 then
curFacingString = west
elseif curFacing == 2 then
curFacingString = south
elseif curFacing == 3 then
curFacingString = east
else
print("Facing direction unknown!")
In this case i get "Facing direction unknown!".
Posted 29 December 2012 - 06:06 AM
I get this weird error, it seems to appear randomly when my turtle moves. I have no clue what it means.
curFacing = 0
curX = 0
curY = 0
curZ = 0
curFacingString = UNKNOWN
function fell()
if turtle.dig() then
turtle.dig()
turtle.forward()
turtle.digUp()
turtle.up()
while turtle.compareUp() == true and turtle.digUp() do
turtle.digUp()
turtle.up()
end
while turtle.down() == true do
turtle.down()
end
end
end
function forward()
turtle.forward()
if curFacing == 0 then
curZ = curZ + 1
elseif curFacing == 1 then
curX = CurX - 1
elseif curFacing == 2 then
curZ = curZ - 1
else
curX = curX + 1
end
saveData()
end
function left()
turtle.turnLeft()
if curFacing == 0 then
curFacing = 3
else
curFacing = curFacing - 1
end
saveData()
end
function right()
turtle.turnRight()
if curFacing == 3 then
curFacing = 0
else
curFacing = curFacing + 1
end
saveData()
end
function up()
turtle.up()
curY = curY + 1
saveData()
end
function down()
turtle.down()
curY = curY - 1
saveData()
end
function saveData()
local file = fs.open("/data", "w")
file.writeLine(curX)
file.writeLine(curY)
file.writeLine(curZ)
file.writeLine(curFacing)
file.close()
end
function loadData()
local file = fs.open("/data", "r")
curX = tonumber(file.readLine())
curY = tonumber(file.readLine())
curZ = tonumber(file.readLine())
curFacing = tonumber(file.readLine())
print("Save data loaded!")
end
function checkTrees()
for i=1, 4, 1 do
forward()
end
left()
for i=1, 3, 1 do
forward()
end
if turtle.compare() then
fell()
left()
forward()
right()
right()
turtle.select(2)
turtle.place()
turtle.select(1)
right()
right()
else
left()
forward()
right()
forward()
left()
end
end
-- Main function
if rs.getInput("right") then
os.terminate()
end
turtle.select(1)
if fs.exists("/data") then
loadData()
else
print("No data found")
end
print(curX.." "..curY.." "..curZ.." "..curFacing)
if turtle.getFuelLevel() >= 200 then
checkTrees()
end
Posted 29 December 2012 - 06:22 AM
Do you still have the testing prints in your fixed code (the ones below that print curX, curY, curZ, and curFacing)? If so, what does it print for curFacing when it says direction is unknown?
Posted 29 December 2012 - 07:32 AM
Do you still have the testing prints in your fixed code (the ones below that print curX, curY, curZ, and curFacing)? If so, what does it print for curFacing when it says direction is unknown?
Anything from 0-3 depending on what it loads from the file, but curFacingString == nil. Also i think i found a way to avoid that code, now i just have this problem: http://www.computercraft.info/forums2/index.php?/topic/7725-luaerror-startup29-attempt-to-perform-arithmetic-sub-on-nil-and-number/
Posted 29 December 2012 - 07:39 AM
Do not create a new post for additional questions about a single piece of code. I will be merging these topics when I get home tonight.
Posted 29 December 2012 - 07:47 AM
Do not create a new post for additional questions about a single piece of code. I will be merging these topics when I get home tonight.
Sorry, I made two threads because it was two different problems I had.
Posted 29 December 2012 - 09:42 AM
curX = CurX - 1
changed that C to lowercase c
Posted 29 December 2012 - 11:54 PM
curX = CurX - 1
changed that C to lowercase c
Oh thanks, that could be it. I really do not understand most of the errors in Lua.
Posted 30 December 2012 - 12:09 AM
CurX = not definied = nil
Posted 30 December 2012 - 12:25 AM
Please make an effort to fix each new problem you encounter before referring it to the forum. While some people take a certain pleasure in fixing errors you haven't even noticed yet, this is not the actual point of this forum.
Posted 30 December 2012 - 01:13 AM
Please make an effort to fix each new problem you encounter before referring it to the forum. While some people take a certain pleasure in fixing errors you haven't even noticed yet, this is not the actual point of this forum.
I do my best to try to fix it myself, but my latest problem was that I didn't know what that error meant, I even looked it up but I didn't find an answer. But now I have learnt what it means, so it won't be a problem in the future I guess. I understand how it is to answer simple questions like that, I do nearly the same on another forum.