Posted 02 January 2013 - 06:36 AM
Hi, I wrote a script that makes the turtle dig 52 blocks down, placing ladders all the way down, then dig a 5x5x4 room. These numbers are all defaults in case tArgs is empty, all of them can be modified by the user. My problem is that when the turtle digs the room at the bottom of the shaft, it is digging an extra row of blocks out of the bottom of the back wall and an extra block out of the bottom of the left wall. Here is a picture in case I am not being clear enough:
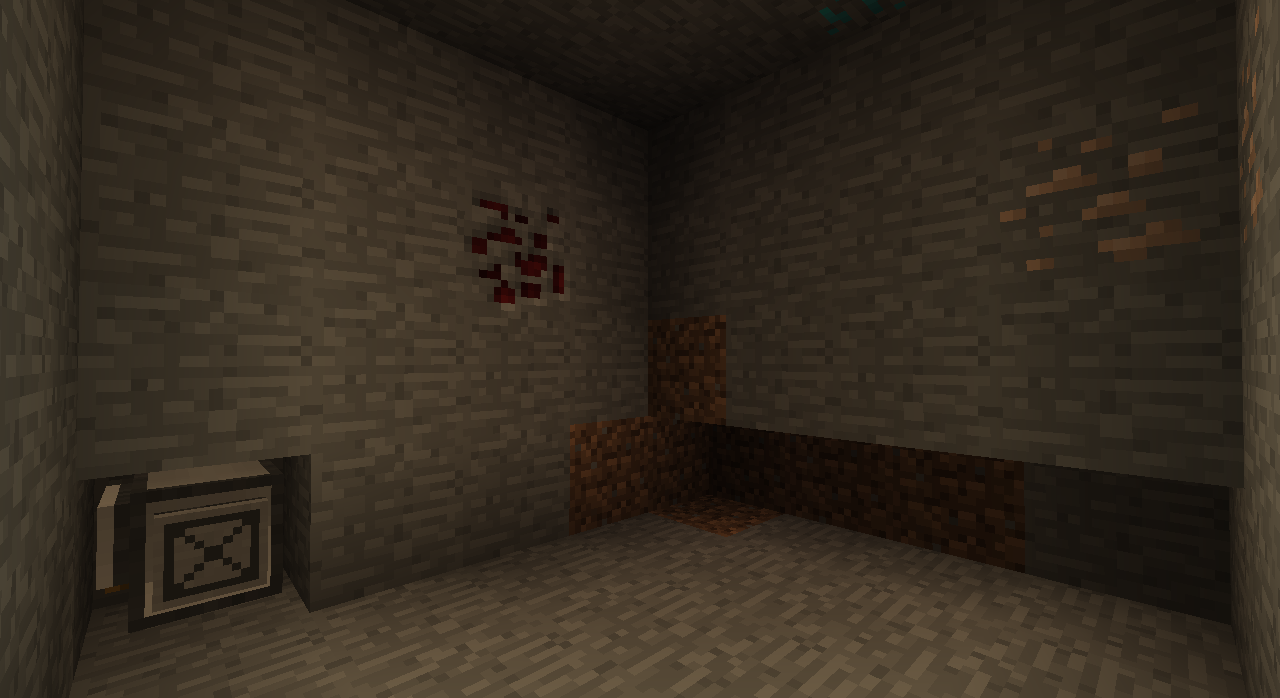
I am 99% sure the problem is with my gravel check in the "smart forward" function I named "fwd()". I just cannot figure out how to make it work without removing the gravel check completely, which would bork my program even worse.
Here is my code, I am pretty new at this, been coding less than a month and never did anything before ComputerCraft, so I am sure it is messy. Any other tips on improving it are welcome and much appreciated, but I really want to figure out how to make the problem described above disappear, as it has been driving me nuts for two days.
Spoiler
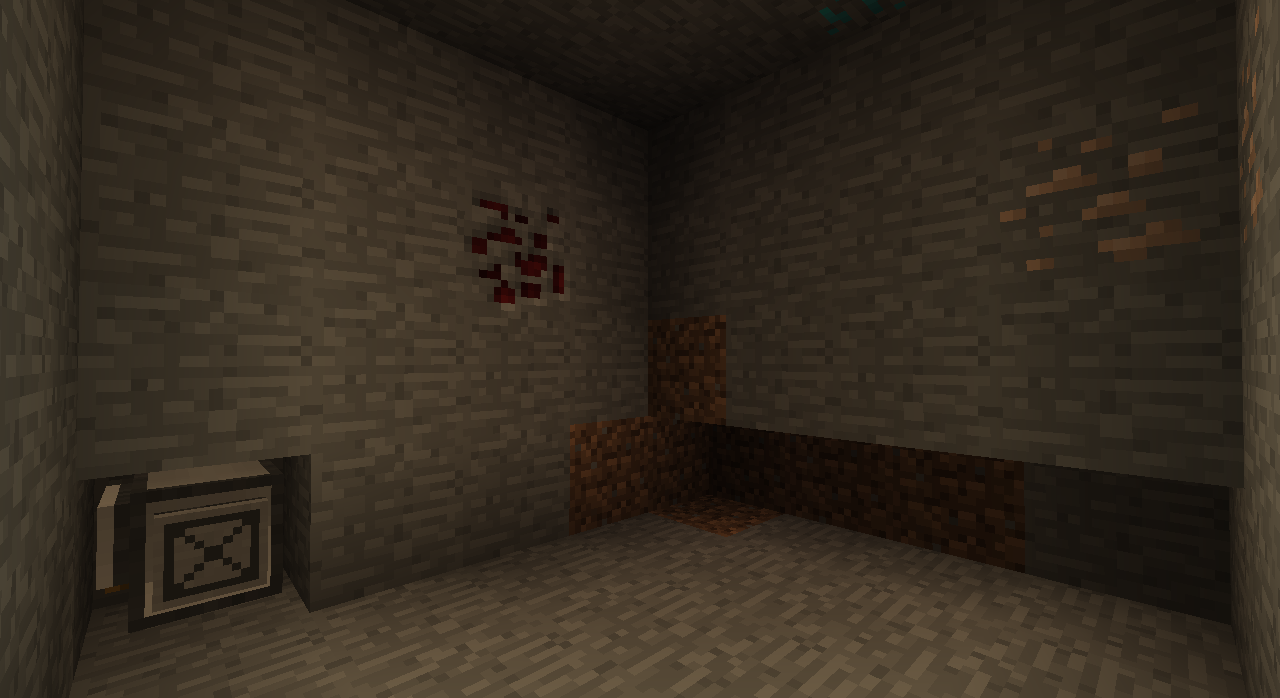
I am 99% sure the problem is with my gravel check in the "smart forward" function I named "fwd()". I just cannot figure out how to make it work without removing the gravel check completely, which would bork my program even worse.
Here is my code, I am pretty new at this, been coding less than a month and never did anything before ComputerCraft, so I am sure it is messy. Any other tips on improving it are welcome and much appreciated, but I really want to figure out how to make the problem described above disappear, as it has been driving me nuts for two days.
Spoiler
--Variables
local tArgs = { ... }
local height = tArgs[1]
local depth = tArgs[2]
local width = tArgs[3]
local ylevel = tArgs[4]
--Functions
local function burrow()
for u = 1, tonumber(ylevel) do
turtle.digDown()
turtle.down()
turtle.select(1)
turtle.placeUp()
end
end
local function fwd() --I think this function is where the problem is.
while not turtle.forward() do
if turtle.detect() then
turtle.dig()
end
end
end
--
local function up()
turtle.digUp()
turtle.up()
end
--
local function left()
turtle.turnLeft()
turtle.dig()
fwd()
turtle.turnRight()
end
--
local function down()
for z = 1, height - 1 do
turtle.digDown()
turtle.down()
end
end
--
local function column()
for a = 1, height - 1 do
up()
end
down()
end
--
local function row()
for b = 1, depth do
column()
fwd()
end
end
--
local function comeHome()
for y = 1, depth do
fwd()
end
end
--
local function right()
turtle.turnRight()
turtle.dig()
fwd()
turtle.turnRight()
end
--
local function wide()
for c = 1, width do
row()
turtle.turnLeft()
turtle.turnLeft()
comeHome()
right()
end
end
--Meat and Potatoes
if (tArgs[1] == nil) then
height = 4
end
if (tArgs[2] == nil) then
depth = 5
end
if (tArgs[3] == nil) then
width = 5
end
if (tArgs[4] == nil) then
ylevel = 52
end
burrow()
fwd()
turtle.digUp()
fwd()
wide()