This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
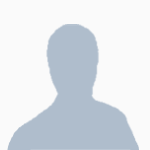
Pausing Certain Loops Only?
Started by nlioc4, 03 January 2013 - 04:31 PMPosted 03 January 2013 - 05:31 PM
I'm trying to run multiple loops at the same time, and when I try and use sleep() inside them, it pauses all loops. Is there any simple way around this? I'll post code if anyone wants it.
Posted 03 January 2013 - 05:33 PM
Code would be great to see how your doing it. If its long please supply it as pastebin. Or else place it in code tags like this ['code] code here ['/code] without the ' of course…
Posted 03 January 2013 - 05:38 PM
function screenWipe()
term.clear()
term.setCursorPos(1, 1)
end
function monitorWipe()
monitor.clear()
monitor.setCursorPos(1,1)
end
function PrintCentered(sText)
local w, h = term.getSize()
local x, y = term.getCursorPos()
x = math.max(math.floor((w / 2) - (#sText / 2)), 0)
term.setCursorPos(x, y)
print(sText)
end
function MonPrintCentered(sText)
local w, h = monitor.getSize()
local x, y = monitor.getCursorPos()
x = math.max(math.floor((w / 2) - (#sText / 2)), 0)
y = y+1
monitor.setCursorPos(x, y)
monitor.write(sText)
end
function AddOutput(sSide, ...)
local c = colors.combine(rs.getBundledOutput(sSide), ...)
rs.setBundledOutput(sSide, c)
end
function RemoveOutput(sSide, ...)
local c = colors.subtract(rs.getBundledOutput(sSide), ...)
rs.setBundledOutput(sSide, c)
end
local function CowTimer()
while true do
AddOutput("back", colors.orange)
RemoveOutput("back", colors.white)
MonPrintCentered("CowKiller Running")
RemoveOutput("back", colors.white)
sleep(5)
AddOutput("back", colors.white)
sleep(295)
RemoveOutput("back", colors.white)
sleep(5)
AddOutput("back", colors.white)
sleep(295)
RemoveOutput("back", colors.white)
sleep(5)
AddOutput("back", colors.white)
sleep(295)
RemoveOutput("back", colors.white)
sleep(5)
AddOutput("back", colors.white)
sleep(295)
RemoveOutput("back", colors.orange)
MonPrintCentered("Water Started")
sleep(20)
AddOutput("back", colors.blue)
MonPrintCentered("Turtles Started")
sleep(1.5)
RemoveOutput("back", colors.blue)
sleep(59.5)
AddOutput("back", colors.orange)
RemoveOutput("back", colors.blue)
sleep(3)
MonPrintCentered("Cow Timer Reseting!")
rednet.broadcast("Farm Control Cow Cycle Completed")
end
end
function ChickenTimer()
while true do
MonPrintCentered("Chicken Killer Running")
MonPrintCentered("Spawning Chickens")
for i=1, 64 do
AddOutput("back", colors.red)
sleep(1)
RemoveOutput("back", colors.red)
end
sleep(1200)
MonPrintCentered("Loading Chickens")
for i=1, 60 do
AddOutput("back", colors.purple)
sleep(0.5)
RemoveOutput("back", colors.purple)
end
sleep(5)
AddOutput("back", colors.yellow)
MonPrintCentered("Killing Chickens")
sleep(5)
RemoveOutput("back", colors.yellow)
MonPrintCentered("Chicken Timer Reseting!")
rednet.broadcast("Farm Control Chicken Cycle Completed")
end
end
CowCorou = coroutine.create(CowTimer)
ChickenCorou = coroutine.create(ChickenTimer)
monitor = peripheral.wrap("top")
rednet.open("bottom")
screenWipe()
monitorWipe()
MonPrintCentered("Farm Control Running")
PrintCentered("Farm Control Running")
monitor.setCursorPos(1,2)
MonPrintCentered("VERY Experimental Version")
coroutine.resume(CowCorou)
coroutine.resume(ChickenCorou)
while true do
print("Would you like to turn wheat production on or off?")
local input = read()
if input == "on" then
AddOutput("back", colors.green)
PrintCentered("Wheat Production Off")
elseif input == "off" then
RemoveOutput("back", colors.green)
PrintCentered("Wheat Production On")
end
end
That's it, it's an incredibly simple timer designed to control an automated chicken and cow farm. I'm just getting the basic controls down at the moment and will add nice interfaces and such later on. What's happening is, the chicken spawner (dispenser filled with eggs) only fires once then stops.
Posted 03 January 2013 - 09:42 PM
if you want to pause certain loops, the most efficient way to do that is coroutine manipulation
turn all the functions into coroutines and put those in a table
keep cycling through the table, resuming the coroutines you want to resume and skipping the ones you want to skip, until you want the program to end or move on
turn all the functions into coroutines and put those in a table
keep cycling through the table, resuming the coroutines you want to resume and skipping the ones you want to skip, until you want the program to end or move on
Posted 03 January 2013 - 09:44 PM
btw, resuming a coroutine pauses the program until the coroutine yields (sleeps), so when you resumed the cow and chicken coroutines, they just went through one cycle
Posted 03 January 2013 - 10:18 PM
Or use parallels and it'll do it for you ;)/>
Posted 04 January 2013 - 02:03 AM
Yeah, I'd just go with this. Easiest and shortest way out.Or use parallels and it'll do it for you ;)/>/>
Posted 04 January 2013 - 12:45 PM
What do you mean by parrallels? I haven't been doing this for very long :P/>
I've been thinking about just downloading some timer api, I think that would work nicely.
I've been thinking about just downloading some timer api, I think that would work nicely.
Posted 04 January 2013 - 01:12 PM
Parallel API is simple.
Prints the words "hello" and "world" ten times at the same time and at random time intervals.
function hello()
for i=1, 10 do
print 'hello'
sleep(math.random())
end
end
function world()
for i=1, 10 do
print 'world'
sleep(math.random())
end
end
parallel.waitForAll(hello, world)
Prints the words "hello" and "world" ten times at the same time and at random time intervals.
Posted 04 January 2013 - 01:59 PM
That is, to say, prints ten each of "hello" and "world", interspersed at random time intervals of up to two seconds (or up to 1+1 seconds, however you want to phrase that).