This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
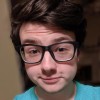
I am making a signin script
Started by ebernerd, 11 January 2013 - 05:25 AMPosted 11 January 2013 - 06:25 AM
So what i want to do is have a default entry and password (default: user=User and password=password) and then you can register in the program, and it will save to a computer either online (when I learn rednet, that would be cool!) or internally in the computer, and I want unlimited entry space, not just one. So "Jim" could logon then logoff and then "Karen" could logon. Could anyone help?
Posted 11 January 2013 - 06:32 AM
You'll want to use a table of username/password pairs stored in a separate file. It'll probably be easiest to manage if you use the username as the key and the password as the value, rather than two numerically-indexed tables.
Posted 11 January 2013 - 06:35 AM
To do this, you could save the usernames and passwords on a file in the computer, and when the user enters their credentials, you could check if it is the same as any of the ones in the file. To add more, you just keep appending to the file. The file could also contain the default username and password.
Example:
Example /credentials file:
Example add username program:
Note: this does not include encryption, salting, or checking if the file exists/contents is valid.
Example:
-- I'll leave the GUI up to you
local enteredUser = "this is the entered username"
local enteredPass = "this is the entered password"
local f = io.open("/credentials", "r")
local available = textutils.unserialize(f:read("*l"))
f:close()
local isCorrect = false
for k, v in pairs(available) do
if enteredUser == k and enteredPass == v then
isCorrect = true
break
end
end
if isCorrect then
-- Login
end
Example /credentials file:
{["Jim"] = "jimspassword", ["Karen"] = "karenspassword"}
Example add username program:
-- I'll leave the GUI up to you
local newUser = "this is the new username"
local newPass = "this is the new password"
local f = io.open("/credentials", "r")
local existing = textutils.unserialize(f:read("*l"))
f:close()
existing[newUser] = newPass
local f = io.open("/credentials", "w")
f:write(textutils.serialize(existing))
f:close()
Note: this does not include encryption, salting, or checking if the file exists/contents is valid.
Posted 11 January 2013 - 06:42 AM
Example /credentials file:{"Jim" = "jimspassword", "Karen" = "karenspassword"}
A minor correction: "Jim" would need to either be Jim or ["Jim"] (I believe textutils.serialize produces the latter); likewise for "Karen".
Posted 11 January 2013 - 06:44 AM
Example /credentials file:{"Jim" = "jimspassword", "Karen" = "karenspassword"}
A minor correction: "Jim" would need to either be Jim or ["Jim"] (I believe textutils.serialize produces the latter); likewise for "Karen".
Ahhh you're right! Thanks Lyqyd. I'll edit the post…
Posted 11 January 2013 - 08:45 AM
So I did this… I added like 10 usernames and random passcodes… will this stay there if I break the computer(I have the credentials on a floppy) or the computer is shut down?To do this, you could save the usernames and passwords on a file in the computer, and when the user enters their credentials, you could check if it is the same as any of the ones in the file. To add more, you just keep appending to the file. The file could also contain the default username and password.
Example:-- I'll leave the GUI up to you local enteredUser = "this is the entered username" local enteredPass = "this is the entered password" local f = io.open("/credentials", "r") local available = textutils.unserialize(f:read("*l")) f:close() local isCorrect = false for k, v in pairs(available) do if enteredUser == k and enteredPass == v then isCorrect = true break end end if isCorrect then -- Login end
Example /credentials file:{["Jim"] = "jimspassword", ["Karen"] = "karenspassword"}
Example add username program:-- I'll leave the GUI up to you local newUser = "this is the new username" local newPass = "this is the new password" local f = io.open("/credentials", "r") local existing = textutils.unserialize(f:read("*l")) f:close() existing[newUser] = newPass local f = io.open("/credentials", "w") f:write(textutils.serialize(existing)) f:close()
Note: this does not include encryption, salting, or checking if the file exists/contents is valid.
Posted 11 January 2013 - 08:50 AM
Actually!To do this, you could save the usernames and passwords on a file in the computer, and when the user enters their credentials, you could check if it is the same as any of the ones in the file. To add more, you just keep appending to the file. The file could also contain the default username and password.
Example:-- I'll leave the GUI up to you local enteredUser = "this is the entered username" local enteredPass = "this is the entered password" local f = io.open("/credentials", "r") local available = textutils.unserialize(f:read("*l")) f:close() local isCorrect = false for k, v in pairs(available) do if enteredUser == k and enteredPass == v then isCorrect = true break end end if isCorrect then -- Login end
Example /credentials file:{["Jim"] = "jimspassword", ["Karen"] = "karenspassword"}
Example add username program:-- I'll leave the GUI up to you local newUser = "this is the new username" local newPass = "this is the new password" local f = io.open("/credentials", "r") local existing = textutils.unserialize(f:read("*l")) f:close() existing[newUser] = newPass local f = io.open("/credentials", "w") f:write(textutils.serialize(existing)) f:close()
Note: this does not include encryption, salting, or checking if the file exists/contents is valid.
I realized(failtest) that Now I cannot add more than one person…. I have tried to change the code.. and it screws it up.
Here is the code for register:
textutils.slowPrint("Registry:")
local newUser = read()
local newPass = read()
local f = io.open("disk/credentials", "r")
local existing = textutils.unserialize(f:read("*l"))
f:close()
existing[newUser] = newPass
local f = io.open("/credentials", "w")
f:write(textutils.serialize(existing))
f:close()
Here is the login:
print("MineOS TEST")
write("Username: ")
local enteredUser = read()
write("Password: ")
local enteredPass = read("*")
local f = io.open("disk/credentials", "r")
local available = textutils.unserialize(f:read("*l"))
f:close()
local isCorrect = false
for k, v in pairs(available) do
if enteredUser == k and enteredPass == v then
isCorrect = true
break
end
end
if isCorrect then
textutils.slowWrite("Validating...")
sleep(1)
textutils.slowWrite("Logging on...")
shell.run("cd disk")
shell.run("clear")
else
textutils.slowPrint("Validating...")
sleep(1)
textutils.slowPrint("Name not in database")
end
I might have the credentials wrong…
Posted 11 January 2013 - 08:52 AM
They should persist across shutdowns regardless, I believe. Labeling the computer will make them persist across breakings. Use the label program: label set <name>
Edit: in the register program, you are reading from disk/credentials and writing the updated list to /credentials. You then read from disk/credentials in the main login program. Updating the register program to write to disk/credentials should fix it.
Edit: in the register program, you are reading from disk/credentials and writing the updated list to /credentials. You then read from disk/credentials in the main login program. Updating the register program to write to disk/credentials should fix it.
Posted 11 January 2013 - 08:52 AM
GOT IT THANKSS>>>>>>>>>>>