- mon = peripheral.wrap("left")
- function lineBreak()
- x, y = mon.getCursorPos()
- if y ~= 1 then
- y = y + 1
- end
- mon.setCursorPos(1, y)
- width, height = mon.getSize()
- mon.write("+" .. string.rep("-", width - 2) .. "+")
- end
- function printString(sString)
- x, y = mon.getCursorPos()
- y = y + 1
- mon.setCursorPos(1, y)
- mon.write(sString)
- end
- function printStringCentre(sString)
- x, y = mon.getCursorPos()
- y = y + 1
- mon.setCursorPos(1, y)
- width, height = mon.getSize()
- nStringCentre = math.floor(string.len(sString) / 2)
- nMonitorCentre = math.floor(width / 2)
- x = math.floor(nMonitorCentre - nStringCentre)
- mon.setCursorPos(x, y)
- mon.write(sString)
- end
- function printStringRight(sString)
- width, height = mon.getSize()
- x, y = mon.getCursorPos()
- y = y + 1
- x = math.ceil(width - string.len(sString))
- mon.setCursorPos(x, y)
- mon.write(sString)
- end
- function scrollText(tStrings, nRate)
- nRate = nRate or 5
- if nRate < 0 then
- error("rate must be positive")
- end
- end
- nSleep = 1 / nRate
- width, height = mon.getSize()
- x, y = mon.getCursorPos()
- sText = ""
- for n = 1, #tStrings do
- sText = sText .. tostring(tStrings[n])
- sText = sText .. " | "
- end
- sString = "| "
- if width / string.len(sText) < 1 then
- nStringRepeat = 3
- else
- nStringRepeat = math.ceil(width / string.len(sText) * 3)
- end
- for n = 1, nStringRepeat do
- sString = sString .. sText
- end
- while true do
- for n = 1, string.len(sText) do
- sDisplay = string.sub(sString, n, n + width - 1)
- mon.clearLine()
- mon.setCursorPos(1, y)
- mon.write(sDisplay)
- sleep(nSleep)
- end
- end
- mon.clear()
- mon.setCursorPos(1, 1)
- lineBreak()
- printStringCentre("|General stuff|")
- lineBreak()
- printString("")
- lineBreak()
- tScrollText = {}
- tScrollText[1] = "Fact:"
- tScrollText[2] = "Rainbowdash is NOT a girl!"
- tScrollText[3] = "Fact:"
- tScrollText[4] = "Grahamcraft is the best server ever in the world"
- lineBreak()
- printStringCentre("Rules")
- lineBreak()
- printString("1, Do not grief**")
- printString("2, Do not disrespect admins*")
- printString("4, Do not lie about donating to the server**")
- printString("5, Do not advertise other servers on ours***")
- printString("6, Do not steal from others**")
- printString("7, No pvp in this world")
- printString("8, Do not ignore these rules, READ THEM NOW!")
- printString("9, Play fair and have fun!***")
- lineBreak()
- printString(" * = warning")
- printString(" ** = depends how serious")
- printString(" *** = Instant ban")
- x, y = mon.getCursorPos()
- y = y - 1
- mon.setCursorPos(1, y)
- scrollText(tScrollText)
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
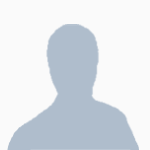
Can Someone tell me what wrong with this program please :(
Started by tommy4eva, 13 January 2013 - 01:52 AMPosted 13 January 2013 - 02:52 AM
It says there is problem with Line 44 attempt to index ? (a nil Value) Can someone please help with this, its essentiall For aa server please reply ASAP, Yours sincerly Tommy4eva.
Posted 13 January 2013 - 03:02 AM
Firstly this is in the wrong section, should be in "Ask a Pro"
Secondly, thank you for putting line numbers, we also have code tags on this forum ;)/> :)/> [code][/code]
Lastly, is this copy pasted or typed out? because i don't see the issue with that line… if it really is that line then getSize() must not be available when using peripheral.wrap… alternatively you could change all the mon. to term. and use term.redirect at the start of the program and term.restore at the end of the program to get it printing on a monitor…
EDIT: Oops just occurred to me that i didn't tell you how to use redirect…
Secondly, thank you for putting line numbers, we also have code tags on this forum ;)/> :)/> [code][/code]
Lastly, is this copy pasted or typed out? because i don't see the issue with that line… if it really is that line then getSize() must not be available when using peripheral.wrap… alternatively you could change all the mon. to term. and use term.redirect at the start of the program and term.restore at the end of the program to get it printing on a monitor…
EDIT: Oops just occurred to me that i didn't tell you how to use redirect…
local mon = peripheral.wrap( "left" )
term.redirect( mon )
-- all your code here
term.restore()
Edited on 13 January 2013 - 02:04 AM
Posted 13 January 2013 - 03:08 AM
thanks but now it says there is a problem with line 44… help
Posted 13 January 2013 - 03:09 AM
that error means you are trying to access something in a table when the table does not actually exist, that code seems fine though as long as mon is not getting overwritten somewhere
Posted 13 January 2013 - 03:16 AM
errm… could you tell me what this means, could you fix it for me, i dont know much about computer craft… :(/>
Posted 13 January 2013 - 03:23 AM
there is no problem with the code above though…. it seems fine. do you have access the the world save folder of the server? if so get the actual program file from there and put it on pastebin, explain what you have set up (where the computer, monitor etc are in the MC world) and I should be able to set up a test world and get it sorted
Posted 13 January 2013 - 03:23 AM
Move line 44 to line 2 so it gets the size of the monitor as the wraps it.
Are you destroying the monitor while the program is running?
Are you destroying the monitor while the program is running?
Posted 13 January 2013 - 03:36 AM
even if you break the monitor you should not get that error, then the peripheral call would just fail
Posted 13 January 2013 - 04:04 AM
What's wrong with this then? (Tommy4evas friend)
I'm clueless about lua btw.
This code worked before but there was a tekkit update and it stops working.
I'm clueless about lua btw.
This code worked before but there was a tekkit update and it stops working.
mon = peripheral.wrap("left")
width, height = mon.getSize()
function lineBreak()
x, y = mon.getCursorPos()
if y ~= 1 then
y = y + 1
end
mon.setCursorPos(1, y)
width, height = mon.getSize()
mon.write("+" .. string.rep("-", width - 2) .. "+")
end
function printString(sString)
x, y = mon.getCursorPos()
y = y + 1
mon.setCursorPos(1, y)
mon.write(sString)
end
function printStringCentre(sString)
x, y = mon.getCursorPos()
y = y + 1
mon.setCursorPos(1, y)
width, height = mon.getSize()
nStringCentre = math.floor(string.len(sString) / 2)
nMonitorCentre = math.floor(width / 2)
x = math.floor(nMonitorCentre - nStringCentre)
mon.setCursorPos(x, y)
mon.write(sString)
end
function printStringRight(sString)
width, height = mon.getSize()
x, y = mon.getCursorPos()
y = y + 1
x = math.ceil(width - string.len(sString))
mon.setCursorPos(x, y)
mon.write(sString)
end
function scrollText(tStrings, nRate)
nRate = nRate or 5
if nRate < 0 then
error("rate must be positive")
end
end
nSleep = nRate
x, y = mon.getCursorPos()
sText = "fds"
for n = 1, #tStrings do
sText = sText .. tostring(tStrings[n])
sText = sText .. " | "
end
sString = "| "
if width / string.len(sText) < 1 then
nStringRepeat = 3
else
nStringRepeat = math.ceil(width / string.len(sText) * 3)
end
for n = 1, nStringRepeat do
sString = sString .. sText
end
while true do
for n = 1, string.len(sText) do
sDisplay = string.sub(sString, n, n + width - 1)
mon.clearLine()
mon.setCursorPos(1, y)
mon.write(sDisplay)
sleep(nSleep)
end
end
mon.clear()
mon.setCursorPos(1, 1)
lineBreak()
printStringCentre("|General stuff|")
lineBreak()
printString("")
lineBreak()
tScrollText = {}
tScrollText[1] = "Fact:"
tScrollText[2] = "Rainbowdash is NOT a girl!"
tScrollText[3] = "Fact:"
tScrollText[4] = "Grahamcraft is the best server ever in the world"
lineBreak()
printStringCentre("Rules")
lineBreak()
printString("1, Do not grief**")
printString("2, Do not disrespect admins*")
printString("4, Do not lie about donating to the server**")
printString("5, Do not advertise other servers on ours***")
printString("6, Do not steal from others**")
printString("7, No pvp in this world")
printString("8, Do not ignore these rules, READ THEM NOW!")
printString("9, Play fair and have fun!***")
lineBreak()
printString(" * = warning")
printString(" ** = depends how serious")
printString(" *** = Instant ban")
x, y = mon.getCursorPos()
y = y - 1
mon.setCursorPos(1, y)
scrollText(tScrollText)
Posted 13 January 2013 - 04:06 AM
error please
Posted 13 January 2013 - 04:09 AM
general:46: attempt to get length of nil
Posted 13 January 2013 - 04:25 AM
Try changing the scrollText function to this?
You had an end in the wrong place causing the function to end after the first if statement
function scrollText(tStrings, nRate)
nRate = nRate or 5
if nRate < 0 then
error("rate must be positive")
end
nSleep = nRate
x, y = mon.getCursorPos()
sText = "fds"
for n = 1, #tStrings do
sText = sText .. tostring(tStrings[n])
sText = sText .. " | "
end
sString = "| "
if width / string.len(sText) < 1 then
nStringRepeat = 3
else
nStringRepeat = math.ceil(width / string.len(sText) * 3)
end
for n = 1, nStringRepeat do
sString = sString .. sText
end
while true do
for n = 1, string.len(sText) do
sDisplay = string.sub(sString, n, n + width - 1)
mon.clearLine()
mon.setCursorPos(1, y)
mon.write(sDisplay)
sleep(nSleep)
end
end
end
You had an end in the wrong place causing the function to end after the first if statement
Posted 13 January 2013 - 04:25 AM
what line is 46…
Posted 13 January 2013 - 06:52 AM
I'm getting a new error now
Here's the current code
Error:
Here's the current code
mon = peripheral.wrap("left")
width, height = mon.getSize()
function lineBreak()
x, y = mon.getCursorPos()
if y ~= 1 then
y = y + 1
end
mon.setCursorPos(1, y)
width, height = mon.getSize()
mon.write("+" .. string.rep("-", width - 2) .. "+")
end
function printString(sString)
x, y = mon.getCursorPos()
y = y + 1
mon.setCursorPos(1, y)
mon.write(sString)
end
function printStringCentre(sString)
x, y = mon.getCursorPos()
y = y + 1
mon.setCursorPos(1, y)
width, height = mon.getSize()
nStringCentre = math.floor(string.len(sString) / 2)
nMonitorCentre = math.floor(width / 2)
x = math.floor(nMonitorCentre - nStringCentre)
mon.setCursorPos(x, y)
mon.write(sString)
end
function printStringRight(sString)
width, height = mon.getSize()
x, y = mon.getCursorPos()
y = y + 1
x = math.ceil(width - string.len(sString))
mon.setCursorPos(x, y)
mon.write(sString)
end
function scrollText(tStrings, nRate)
nRate = nRate or 5
if nRate < 0 then
error("rate must be positive")
end
nSleep = nRate
x, y = mon.getCursorPos()
sText = "fds"
for n = 1, #tStrings do
sText = sText .. tostring(tStrings[n])
sText = sText .. " | "
end
sString = "| "
if width / string.len(sText) < 1 then
nStringRepeat = 3
else
nStringRepeat = math.ceil(width / string.len(sText) * 3)
end
for n = 1, nStringRepeat do
sString = sString .. sText
end
while true do
for n = 1, string.len(sText) do
sDisplay = string.sub(sString, n, n + width - 1)
mon.clearLine()
mon.setCursorPos(1, y)
mon.write(sDisplay)
sleep(nSleep)
end
end
end
mon.clear()
mon.setCursorPos(1, 1)
lineBreak()
printStringCentre("|General stuff|")
lineBreak()
printString("")
lineBreak()
tScrollText = {}
tScrollText[1] = "Fact:"
tScrollText[2] = "R"
tScrollText[3] = "Fact:"
tScrollText[4] = "G"
lineBreak()
printStringCentre("Rules")
lineBreak()
printString("1,")
printString("2,")
printString("4,")
printString("5,")
printString("6,")
printString("7,")
printString("8,")
printString("9,")
lineBreak()
printString("g")
printString("s")
printString("h")
x, y = mon.getCursorPos()
y = y - 1
mon.setCursorPos(1, y)
scrollText(tScrollText)
Error:
general:2:attempt to call index ?(a nil value)
Posted 13 January 2013 - 06:57 AM
I just ran the program and it works fine…the only reason you would get that error is if there isn't a monitor on the left side
Posted 13 January 2013 - 07:04 AM
Ah that would make sense, thanks.
I'm being a bit stupid today :P/>
I'm being a bit stupid today :P/>
Posted 13 January 2013 - 07:14 AM
its fine…just make sure your using monitors not computers :P/>