This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
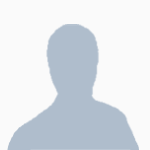
[Solved] Keyboard Inputs
Started by TipTricky, 18 January 2013 - 05:26 PMPosted 18 January 2013 - 06:26 PM
I was wondering how you get keyboard inputs in a program. Like lets say i ran a program i made that needs some one to type in a password to get it to run how would i get the key board inputs.
Posted 18 January 2013 - 06:35 PM
There are a couple of ways, to just capture user input try read() or io.read()
write("Enter Password: ")
local password = read("*") -- the "*" makes the text appear as * on the screen
Posted 18 January 2013 - 06:45 PM
Ok so is io.read() better to use in this case or os.pullEvent()?
io.read() seems simpler than os.pullEvent() correct?
io.read() seems simpler than os.pullEvent() correct?
Posted 18 January 2013 - 06:46 PM
it is much simpler. however it will only read ascii chars. if you wish to read other keys such as enter or control you will need to use an event loop.
Posted 18 January 2013 - 06:50 PM
Thank you for the help read() work perfectly.
Posted 18 January 2013 - 11:19 PM
you could do on an if statement:
x = read("*")
if x == "passcode" then
--code
Posted 19 January 2013 - 12:48 AM
write("Password: ")
local input = read("*")
local pass = "123" --Password
if input == pass then
print("Welcome")
elseif input ~= pass then
print("Wrong password")
else
print("Unknown error")
end
Posted 20 January 2013 - 02:11 AM
(taken down by owner)
Posted 20 January 2013 - 02:19 AM
This looks fine. however you don't need that second elseif doing this will do the exact same thingwrite("Password: ") local input = read("*") local pass = "123" --Password if input == pass then print("Welcome") elseif input ~= pass then print("Wrong password") else print("Unknown error") end
if input == pass then
-- code when they are equivalent
else
-- code when that are not equivalent
end
Also you may want to surround the entire thing with an infinite while loop so that the program will not exit after they have pressed enter…
while true do
-- program code here
end
The "Ask a Pro" section isn't here for advertising. Its here to help people.Or just use my lock it has custom pass input
Posted 20 January 2013 - 12:01 PM
sorryThis looks fine. however you don't need that second elseif doing this will do the exact same thingwrite("Password: ") local input = read("*") local pass = "123" --Password if input == pass then print("Welcome") elseif input ~= pass then print("Wrong password") else print("Unknown error") end
Also you may want to surround the entire thing with an infinite while loop so that the program will not exit after they have pressed enter…if input == pass then -- code when they are equivalent else -- code when that are not equivalent end
while true do -- program code here end
The "Ask a Pro" section isn't here for advertising. Its here to help people.Or just use my lock it has custom pass input